На форму нужно добавить ScriptManager, который регистрирует JavaScript файлы и функции, которые необходимы для реализации AJAX запросов.
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title>New Страница</title> <style> #main { height: 300px; width: 950px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -475px; border-radius: 25px; background-color: #cde3fb; } .wrapper { height: 100%; width: 20%; float: left; } .a { height: 30px; width: 150px; position: relative; top: 50%; left: 50%; margin-top: -15px; margin-left: -75px; text-align: center; line-height: 30px; font-size: 16px; } #Button1 { cursor: pointer; height: 35px; } .error { color: #F00; border: 1px solid #F00; font-size: 12px; } </style> </head> <body> <form id="form1" runat="server"> <%-- добавляем ScriptManager --%> <%-- добавляем атрибут EnablePartialRendering="true", который указывает на то, что фрагменты страницы будут обновляться независимо --%> <asp:ScriptManager ID="ScriptManager1" runat="server" EnablePartialRendering="true"></asp:ScriptManager> <div> <%-- добавляем UpdatePanel --%> <%-- добавляем атрибут UpdateMode="Conditional", который указывает на то, что обновление UpdatePanel будет зависеть от некоторых условий --%> <%-- добавляем атрибут ChildrenAsTriggers="true", который указывает на то, что события элементов управления, расположенных внутри UpdatePanel должны будут вызываться при обращении к серверу --%> <asp:UpdatePanel ID="UpdatePanel1" runat="server" UpdateMode="Conditional" ChildrenAsTriggers="true"> <%-- добавляем секцию ContentTemplate, в нее помещаем контент, который должен обновляться независимо --%> <ContentTemplate> <div id="main"> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox1" runat="server"></asp:TextBox> </div> <div class="wrapper"> <asp:DropDownList CssClass="a" ID="DropDownList1" runat="server"> <asp:ListItem></asp:ListItem> <asp:ListItem>+</asp:ListItem> <asp:ListItem>-</asp:ListItem> <asp:ListItem>*</asp:ListItem> <asp:ListItem>/</asp:ListItem> <asp:ListItem>%</asp:ListItem> </asp:DropDownList> </div> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox2" runat="server"></asp:TextBox> </div> <div class="wrapper"> <asp:Button CssClass="a" ID="Button1" runat="server" Text="=" OnClick="Button1_Click" /> </div> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox3" runat="server" ReadOnly="True"></asp:TextBox> </div> </div> </ContentTemplate> </asp:UpdatePanel> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title>New Страница</title> <style> #main { height: 300px; width: 950px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -475px; border-radius: 25px; background-color: #cde3fb; } .wrapper { height: 100%; width: 20%; float: left; } .a { height: 30px; width: 150px; position: relative; top: 50%; left: 50%; margin-top: -15px; margin-left: -75px; text-align: center; line-height: 30px; font-size: 16px; } #Button1 { cursor: pointer; height: 35px; } .error { color: #F00; border: 1px solid #F00; font-size: 12px; } </style> </head> <body> <form id="form1" runat="server"> <%-- добавляем ScriptManager --%> <%-- добавляем атрибут EnablePartialRendering="true", который указывает на то, что фрагменты страницы будут обновляться независимо --%> <asp:ScriptManager ID="ScriptManager1" runat="server" EnablePartialRendering="true"></asp:ScriptManager> <div> <%-- добавляем UpdatePanel --%> <%-- добавляем атрибут UpdateMode="Conditional", который указывает на то, что обновление UpdatePanel будет зависеть от некоторых условий --%> <%-- добавляем атрибут ChildrenAsTriggers="true", который указывает на то, что события элементов управления, расположенных внутри UpdatePanel должны будут вызываться при обращении к серверу --%> <asp:UpdatePanel ID="UpdatePanel1" runat="server" UpdateMode="Conditional" ChildrenAsTriggers="true"> <%-- добавляем секцию ContentTemplate, в нее помещаем контент, который должен обновляться независимо --%> <ContentTemplate> <div id="main"> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox1" runat="server"></asp:TextBox> </div> <div class="wrapper"> <asp:DropDownList CssClass="a" ID="DropDownList1" runat="server"> <asp:ListItem></asp:ListItem> <asp:ListItem>+</asp:ListItem> <asp:ListItem>-</asp:ListItem> <asp:ListItem>*</asp:ListItem> <asp:ListItem>/</asp:ListItem> <asp:ListItem>%</asp:ListItem> </asp:DropDownList> </div> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox2" runat="server"></asp:TextBox> </div> <div class="wrapper"> <asp:Button CssClass="a" ID="Button1" runat="server" Text="=" OnClick="Button1_Click" /> </div> <div class="wrapper"> <asp:TextBox CssClass="a" ID="TextBox3" runat="server" ReadOnly="True"></asp:TextBox> </div> </div> </ContentTemplate> </asp:UpdatePanel> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { if((sender as Button).Text == "=") { //изменяем текст кнопки (sender as Button).Text = "Clean"; switch(DropDownList1.Text) { case "": TextBox3.Text = string.Empty; break; case "+": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) + decimal.Parse(TextBox2.Text))).ToString(); break; case "-": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) - decimal.Parse(TextBox2.Text))).ToString(); break; case "*": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) * decimal.Parse(TextBox2.Text))).ToString(); break; case "/": if(TextBox2.Text == "0") { TextBox3.CssClass = "a error"; TextBox3.Text = "На ноль делить нельзя!"; } else { TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) / decimal.Parse(TextBox2.Text))).ToString(); } break; case "%": if(TextBox2.Text == "0") { TextBox3.CssClass = "a error"; TextBox3.Text = "На ноль делить нельзя!"; } else { TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) % decimal.Parse(TextBox2.Text))).ToString(); } break; } } else { //изменяем текст кнопки (sender as Button).Text = "="; TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; DropDownList1.ClearSelection(); TextBox3.CssClass = "a"; } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { if((sender as Button).Text == "=") { //изменяем текст кнопки (sender as Button).Text = "Clean"; switch(DropDownList1.Text) { case "": TextBox3.Text = string.Empty; break; case "+": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) + decimal.Parse(TextBox2.Text))).ToString(); break; case "-": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) - decimal.Parse(TextBox2.Text))).ToString(); break; case "*": TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) * decimal.Parse(TextBox2.Text))).ToString(); break; case "/": if(TextBox2.Text == "0") { TextBox3.CssClass = "a error"; TextBox3.Text = "На ноль делить нельзя!"; } else { TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) / decimal.Parse(TextBox2.Text))).ToString(); } break; case "%": if(TextBox2.Text == "0") { TextBox3.CssClass = "a error"; TextBox3.Text = "На ноль делить нельзя!"; } else { TextBox3.Text = ((decimal)(decimal.Parse(TextBox1.Text) % decimal.Parse(TextBox2.Text))).ToString(); } break; } } else { //изменяем текст кнопки (sender as Button).Text = "="; TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; DropDownList1.ClearSelection(); TextBox3.CssClass = "a"; } } }
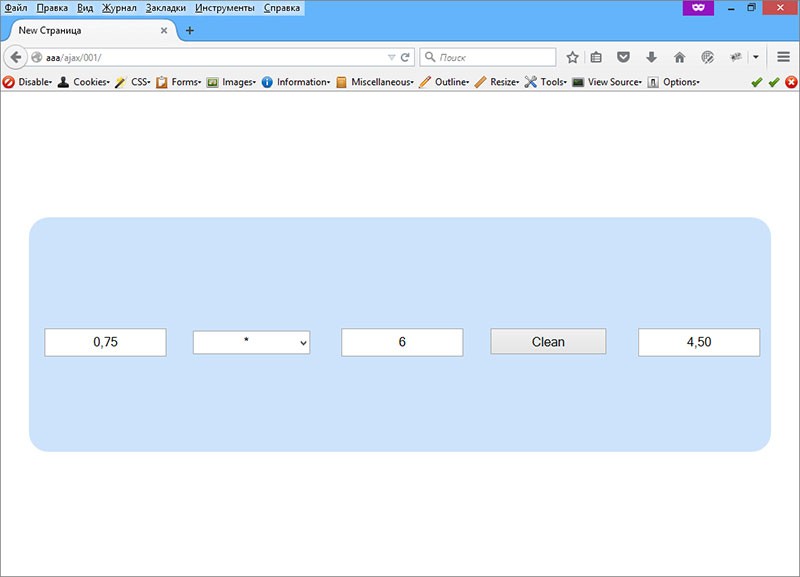