В этом примере из файла index.html будут переданы данные в виде объекта (или в виде строки или в виде массива объектов) на сервер, в файл a.php. Там, эти данные будут обработаны и возвращены в файл index.html.
В файле b.php будет сгенерировано серверное время. С помощью ajax запроса это время будет записано в блок с идентификатором time. Функция setInterval() будет выполнять каждую секунду функцию, выполняющую такой запрос.
В файле b.php будет сгенерировано серверное время. С помощью ajax запроса это время будет записано в блок с идентификатором time. Функция setInterval() будет выполнять каждую секунду функцию, выполняющую такой запрос.
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>New Страница</title> <!-- подключаем библиотеку онлайн через Google CDN --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> <style> #main { height: 298px; width: 948px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -475px; border-radius: 25px; background-color: #cde3fb; border: 1px solid #808080; } .wrapper { height: 100%; width: 20%; float: left; } .a { height: 30px; width: 150px; position: relative; top: 50%; left: 50%; margin-top: -15px; margin-left: -75px; text-align: center; line-height: 30px; font-size: 16px; } #button { cursor: pointer; background-color: #eeeeee; border-radius: 3px; display: inline-block; border: 1px solid #808080; font-size: 18px; } .error { color: #F00; border: 1px solid #F00; font-size: 12px; } #time { margin: 20px; font-size: 36px; color: #6b0698; } </style> </head> <body> <div id="time"></div> <form id="main" action="a.php" method="post"> <div class="wrapper"> <input class="a" type="text" name="inputOne" /> </div> <div class="wrapper"> <select class="a" name="selectChoose"> <option value=""></option> <option value="+">+</option> <option value="-">-</option> <option value="*">*</option> <option value="/">/</option> <option value="%">%</option> </select> </div> <div class="wrapper"> <input class="a" type="text" name="inputTwo" /> </div> <div class="wrapper"> <div class="a" id="button">=</div> </div> <div class="wrapper"> <input id="res" class="a" type="text" name="inputResult" readonly="readonly" /> </div> </form> <script> /*время*/ function funA() { $.ajax({ url: 'b.php', dataType: 'json', cache: false, success: function (str) { $('#time').text(str[0]); } }); } $(document).ready(function () { /*время*/ setInterval('funA()', 1000); //счетчик var counter = 0; $('#button').click(function () { counter++; var strInForm = $('form').serialize(); //нажатие на кнопку 1, 3, 5 и т.д. if(counter % 2 != 0) { $(this).text('Clean'); $.ajax({ url: 'a.php', cache: false, type: 'POST', dataType: 'json', data: strInForm, success: function (strFromForm) { $('#main').css({ 'border': '1px solid #00F' }); $('[name=inputResult]').val(strFromForm[0]); if($('[name=inputResult]').val() == 'На ноль делить нельзя!') { $('[name=inputResult]').addClass('error'); } else { $('[name=inputResult]').removeClass('error'); } }, error: function () { $('#main').css({ 'border': '1px solid #F00' }); } }); } //нажатие на кнопку 2, 4, 6 и т.д. else { $(this).text('='); $('input').val(null); $('select').val(null); $('[name=inputResult]').removeClass('error'); } }); }); </script> </body> </html>
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>New Страница</title> <!-- подключаем библиотеку онлайн через Google CDN --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> <style> #main { height: 298px; width: 948px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -475px; border-radius: 25px; background-color: #cde3fb; border: 1px solid #808080; } .wrapper { height: 100%; width: 20%; float: left; } .a { height: 30px; width: 150px; position: relative; top: 50%; left: 50%; margin-top: -15px; margin-left: -75px; text-align: center; line-height: 30px; font-size: 16px; } #button { cursor: pointer; background-color: #eeeeee; border-radius: 3px; display: inline-block; border: 1px solid #808080; font-size: 18px; } .error { color: #F00; border: 1px solid #F00; font-size: 12px; } #time { margin: 20px; font-size: 36px; color: #6b0698; } </style> </head> <body> <div id="time"></div> <form id="main" action="a.php" method="post"> <div class="wrapper"> <input class="a" type="text" name="inputOne" /> </div> <div class="wrapper"> <select class="a" name="selectChoose"> <option value=""></option> <option value="+">+</option> <option value="-">-</option> <option value="*">*</option> <option value="/">/</option> <option value="%">%</option> </select> </div> <div class="wrapper"> <input class="a" type="text" name="inputTwo" /> </div> <div class="wrapper"> <div class="a" id="button">=</div> </div> <div class="wrapper"> <input id="res" class="a" type="text" name="inputResult" readonly="readonly" /> </div> </form> <script> /*время*/ function funA() { $.ajax({ url: 'b.php', dataType: 'json', cache: false, success: function (str) { $('#time').text(str[0]); } }); } $(document).ready(function () { /*время*/ setInterval('funA()', 1000); //счетчик var counter = 0; $('#button').click(function () { counter++; var strInForm = $('form').serialize(); //нажатие на кнопку 1, 3, 5 и т.д. if(counter % 2 != 0) { $(this).text('Clean'); $.ajax({ url: 'a.php', cache: false, type: 'POST', dataType: 'json', data: strInForm, success: function (strFromForm) { $('#main').css({ 'border': '1px solid #00F' }); $('[name=inputResult]').val(strFromForm[0]); if($('[name=inputResult]').val() == 'На ноль делить нельзя!') { $('[name=inputResult]').addClass('error'); } else { $('[name=inputResult]').removeClass('error'); } }, error: function () { $('#main').css({ 'border': '1px solid #F00' }); } }); } //нажатие на кнопку 2, 4, 6 и т.д. else { $(this).text('='); $('input').val(null); $('select').val(null); $('[name=inputResult]').removeClass('error'); } }); }); </script> </body> </html>
a.php
<?PHP header('Content-Type: text/html; charset=utf-8'); class Calculation { private $inputOne; private $selectChoose; private $inputTwo; function __construct($arg0, $arg1, $arg2) { $this->inputOne = floatval($arg0); $this->selectChoose = $arg1; $this->inputTwo = floatval($arg2); } function Result() { switch($this->selectChoose) { case '': return ''; break; case '+': return $this->inputOne + $this->inputTwo; break; case '-': return $this->inputOne - $this->inputTwo; break; case '*': return $this->inputOne * $this->inputTwo; break; case '/': if((int)$this->inputTwo == 0) { return 'На ноль делить нельзя!'; } else { return $this->inputOne / $this->inputTwo; } break; case '%': if((int)$this->inputTwo == 0) { return 'На ноль делить нельзя!'; } else { return (int)$this->inputOne % (int)$this->inputTwo; } break; } } } $C = new Calculation($_POST['inputOne'], $_POST['selectChoose'], $_POST['inputTwo']); $ara = array($C->Result()); $str = json_encode($ara); echo $str; ?>
<?PHP header('Content-Type: text/html; charset=utf-8'); class Calculation { private $inputOne; private $selectChoose; private $inputTwo; function __construct($arg0, $arg1, $arg2) { $this->inputOne = floatval($arg0); $this->selectChoose = $arg1; $this->inputTwo = floatval($arg2); } function Result() { switch($this->selectChoose) { case '': return ''; break; case '+': return $this->inputOne + $this->inputTwo; break; case '-': return $this->inputOne - $this->inputTwo; break; case '*': return $this->inputOne * $this->inputTwo; break; case '/': if((int)$this->inputTwo == 0) { return 'На ноль делить нельзя!'; } else { return $this->inputOne / $this->inputTwo; } break; case '%': if((int)$this->inputTwo == 0) { return 'На ноль делить нельзя!'; } else { return (int)$this->inputOne % (int)$this->inputTwo; } break; } } } $C = new Calculation($_POST['inputOne'], $_POST['selectChoose'], $_POST['inputTwo']); $ara = array($C->Result()); $str = json_encode($ara); echo $str; ?>
b.php
<?PHP header('Content-Type: text/html; charset=utf-8'); $ara = array(date('H:i:s')); $str = json_encode($ara); echo $str; ?>
<?PHP header('Content-Type: text/html; charset=utf-8'); $ara = array(date('H:i:s')); $str = json_encode($ara); echo $str; ?>
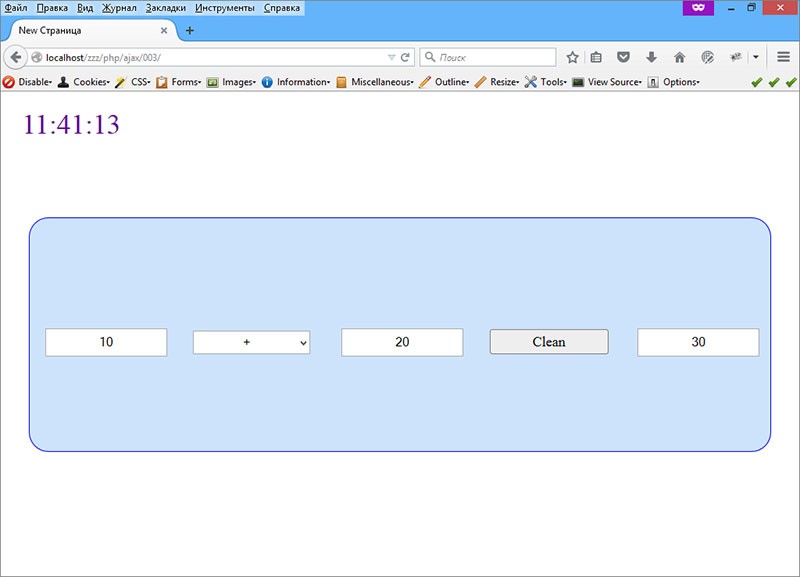