В этом примере, пользователь вычисляет в дочерней форме расчет за электроэнергию, сумма одновременно отображается и в дочерней форме и в родительской. Точно так же и с расчетом за воду. В родительской форме эти суммы одновременно складываются.
Counter.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0020 {
-
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0020 {
-
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
-
}
}
GeneralSum.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0020 {
-
class
GeneralSum {
-
public
static
decimal
Add(
params
string
[] args) {
-
decimal
res = 0;
-
for
(
int
i=0; i<args.Length; i++) {
-
decimal
val;
-
decimal
.TryParse(args[i],
out
val);
-
res += val;
-
}
-
return
Math.Round(res, 2);
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0020 {
-
class
GeneralSum {
-
public
static
decimal
Add(
params
string
[] args) {
-
decimal
res = 0;
-
for
(
int
i=0; i<args.Length; i++) {
-
decimal
val;
-
decimal
.TryParse(args[i],
out
val);
-
res += val;
-
}
-
return
Math.Round(res, 2);
-
}
-
}
}
Form2Elcectro.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form2Elcectro : Form {
-
public
Form2Elcectro() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
//создаем переменную класса Form1 и присваиваем ей значение через ссылку
-
Form1 F1 = (Form1)
this
.Owner;
-
//текстовому полю родительской формы присваиваем значение суммы через свойство
-
F1.TextBox1SumElectro.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form2Elcectro : Form {
-
public
Form2Elcectro() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
//создаем переменную класса Form1 и присваиваем ей значение через ссылку
-
Form1 F1 = (Form1)
this
.Owner;
-
//текстовому полю родительской формы присваиваем значение суммы через свойство
-
F1.TextBox1SumElectro.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
Form3Water.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form3Water : Form {
-
public
Form3Water() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
//создаем переменную класса Form1 и присваиваем ей значение через ссылку
-
Form1 F1 = (Form1)
this
.Owner;
-
//текстовому полю родительской формы присваиваем значение суммы через свойство
-
F1.TextBox1SumWater.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form3Water : Form {
-
public
Form3Water() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
//создаем переменную класса Form1 и присваиваем ей значение через ссылку
-
Form1 F1 = (Form1)
this
.Owner;
-
//текстовому полю родительской формы присваиваем значение суммы через свойство
-
F1.TextBox1SumWater.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
Form1.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
//создаем свойство для доступа к полю из дочерней формы через ссылку
-
public
TextBox TextBox1SumElectro {
-
set
{
-
textBox1 = value;
-
}
-
get
{
-
return
textBox1;
-
}
-
}
-
//создаем свойство для доступа к полю из дочерней формы через ссылку
-
public
TextBox TextBox1SumWater {
-
set
{
-
textBox2 = value;
-
}
-
get
{
-
return
textBox2;
-
}
-
}
-
private
void
Form1_Activated(
object
sender, EventArgs e) {
-
//при активации формы вычисляется общая сумма
-
textBox3.Text = GeneralSum.Add(textBox1.Text, textBox2.Text).ToString();
-
}
-
private
void
button3_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//открываем дочернюю форму и передаем ей ссылку
-
new
Form2Elcectro().ShowDialog(
this
);
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//открываем дочернюю форму и передаем ей ссылку
-
new
Form3Water().ShowDialog(
this
);
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0020 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
//создаем свойство для доступа к полю из дочерней формы через ссылку
-
public
TextBox TextBox1SumElectro {
-
set
{
-
textBox1 = value;
-
}
-
get
{
-
return
textBox1;
-
}
-
}
-
//создаем свойство для доступа к полю из дочерней формы через ссылку
-
public
TextBox TextBox1SumWater {
-
set
{
-
textBox2 = value;
-
}
-
get
{
-
return
textBox2;
-
}
-
}
-
private
void
Form1_Activated(
object
sender, EventArgs e) {
-
//при активации формы вычисляется общая сумма
-
textBox3.Text = GeneralSum.Add(textBox1.Text, textBox2.Text).ToString();
-
}
-
private
void
button3_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//открываем дочернюю форму и передаем ей ссылку
-
new
Form2Elcectro().ShowDialog(
this
);
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//открываем дочернюю форму и передаем ей ссылку
-
new
Form3Water().ShowDialog(
this
);
-
}
-
}
}
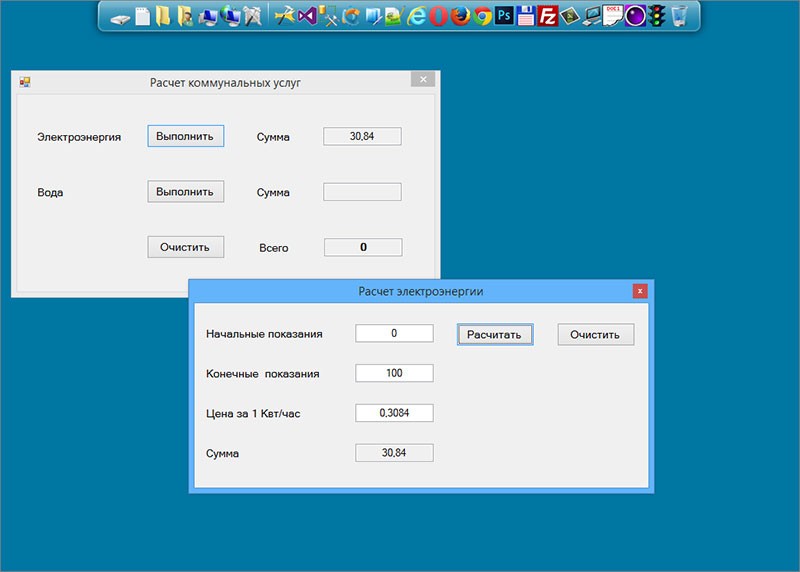
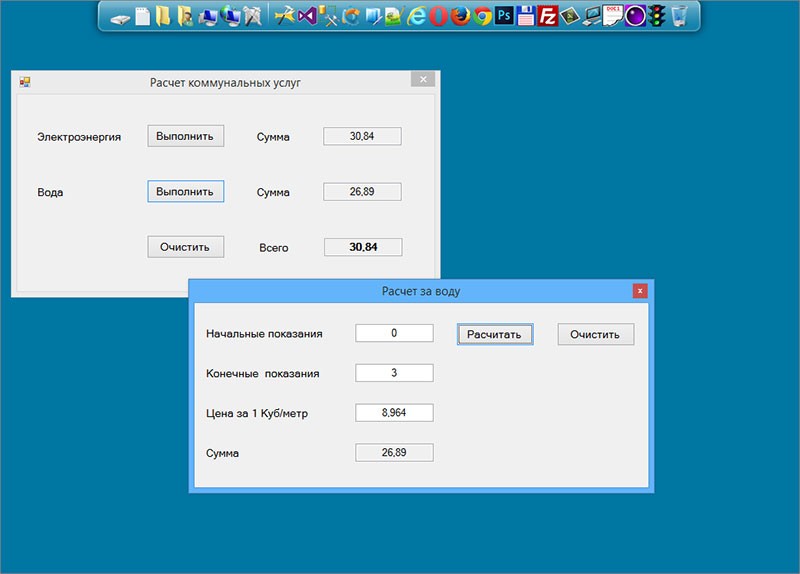
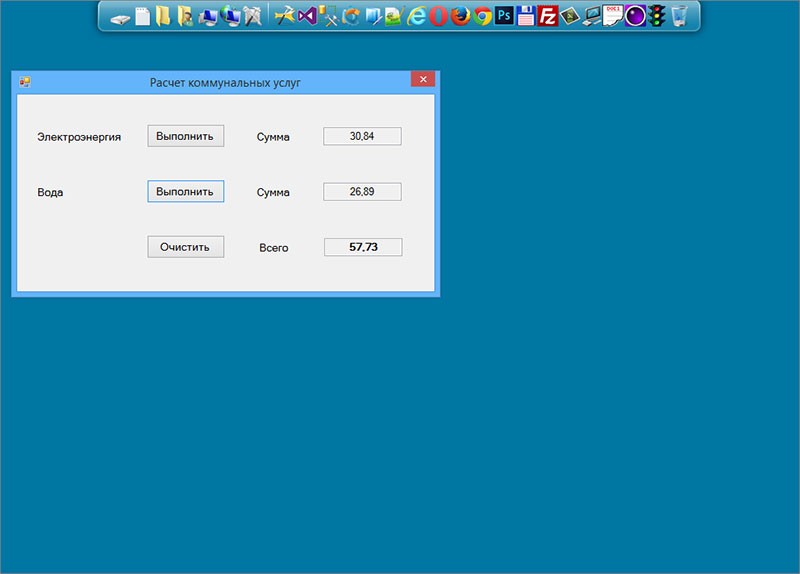