В дочерней форме создаем свойство, которому будет присвоено значение из родительской формы.
В родительской форме присваиваем значение полю.
Передать данные можно только один раз, в момент создания дочерней формы.
В родительской форме присваиваем значение полю.
Передать данные можно только один раз, в момент создания дочерней формы.
Counter.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0022 {
-
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
_0022 {
-
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
-
}
}
Form2Child.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0022 {
-
public
partial
class
Form2Child : Form {
-
//создаем свойство, которому будет присвоено значение из родительской формы
-
public
TextBox TextBox3Price {
-
set
{
-
textBox3 = value;
-
}
-
get
{
-
return
textBox3;
-
}
-
}
-
public
Form2Child() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0022 {
-
public
partial
class
Form2Child : Form {
-
//создаем свойство, которому будет присвоено значение из родительской формы
-
public
TextBox TextBox3Price {
-
set
{
-
textBox3 = value;
-
}
-
get
{
-
return
textBox3;
-
}
-
}
-
public
Form2Child() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
//вычисляем сумму
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
}
-
}
}
Form1.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0022 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
Form2Child F2 =
new
Form2Child();
-
//присваиваем значение свойству дочерней формы
-
F2.TextBox3Price.Text = textBox1.Text;
-
//вызываем дочернюю форму
-
F2.ShowDialog();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
textBox1.Clear();
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0022 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
Form2Child F2 =
new
Form2Child();
-
//присваиваем значение свойству дочерней формы
-
F2.TextBox3Price.Text = textBox1.Text;
-
//вызываем дочернюю форму
-
F2.ShowDialog();
-
}
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка Очистить очищает все текстовые поля
-
textBox1.Clear();
-
}
-
}
}
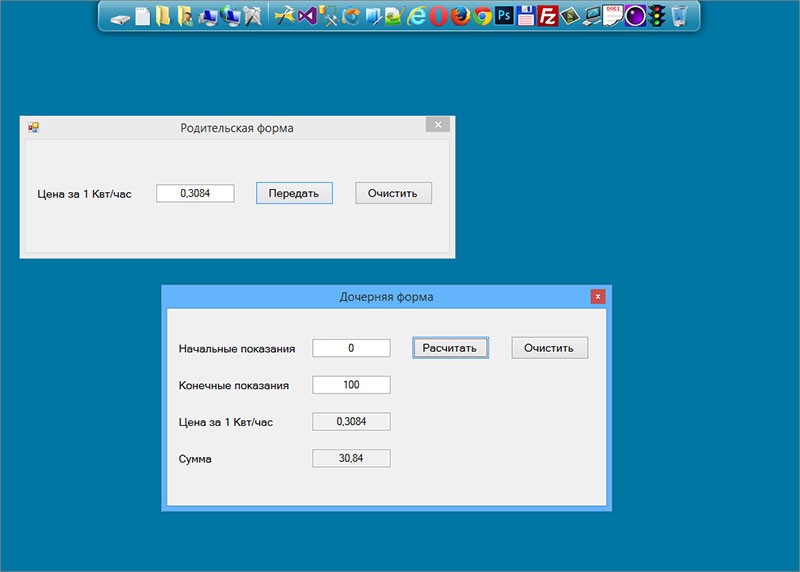