В предыдущем примере было показано, как с помощью нажатия клавиши, например Enter, переходить к нужному элементу. Раздражение вызывал системный звук. В этом примере мы удалим этот звук.
Текстовое поле имеет особенность издавать системный звук, при нажатии клавиши, когда оно в фокусе.
Что бы убрать звук, нужно перехватить нажатие этой клавиши.
Текстовое поле имеет особенность издавать системный звук, при нажатии клавиши, когда оно в фокусе.
Что бы убрать звук, нужно перехватить нажатие этой клавиши.
Counter.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
class
Counter {
-
ushort
showStart;
-
ushort
showEnd;
-
decimal
price;
-
public
Counter(
string
arg0,
string
arg1,
string
arg2) {
-
ushort
.TryParse(arg0,
out
showStart);
-
ushort
.TryParse(arg1,
out
showEnd);
-
decimal
.TryParse(arg2,
out
price);
-
}
-
public
ushort
ResultShow() {
-
return
(
ushort
)(showEnd - showStart);
-
}
-
public
decimal
Sum() {
-
return
Math.Round((
decimal
)(ResultShow() * price) , 2);
-
}
}
Form1.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0027 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
//кнопка Расчитать вычисляет сумму и активирует текстовое поле Сумма
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
textBox4.Select();
-
//textBox4.Focus();
-
}
-
//кнопка Очистить очищает все текстовые поля и активирует текстовое поле Начальные показания
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
textBox1.Select();
-
//textBox1.Focus();
-
}
-
//текстовое поле Начальные показания активирует текстовое поле Конечные показания
-
private
void
textBox1_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
textBox2.Select();
-
//textBox2.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Конечные показания активирует текстовое поле Цена за 1 Квт/час
-
private
void
textBox2_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
textBox3.Select();
-
//textBox3.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Цена за 1 Квт/час активирует кнопку Расчитать
-
private
void
textBox3_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
button1.Select();
-
//button1.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Сумма активирует кнопку Очистить
-
private
void
textBox4_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
button2.Select();
-
//button2.Focus();
-
//если в текстовом поле свойство ReadOnly = true, делать перехват клавиши не обязательно
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0027 {
-
public
partial
class
Form1 : Form {
-
public
Form1() {
-
InitializeComponent();
-
}
-
//кнопка Расчитать вычисляет сумму и активирует текстовое поле Сумма
-
private
void
button1_Click(
object
sender, EventArgs e) {
-
Counter C =
new
Counter(textBox1.Text, textBox2.Text, textBox3.Text);
-
textBox4.Text = C.Sum().ToString();
-
textBox4.Select();
-
//textBox4.Focus();
-
}
-
//кнопка Очистить очищает все текстовые поля и активирует текстовое поле Начальные показания
-
private
void
button2_Click(
object
sender, EventArgs e) {
-
//кнопка очищает все текстовые поля
-
foreach
(Control i
in
Controls) {
-
if
(i.GetType() ==
typeof
(TextBox)) {
-
i.Text =
string
.Empty;
-
}
-
}
-
textBox1.Select();
-
//textBox1.Focus();
-
}
-
//текстовое поле Начальные показания активирует текстовое поле Конечные показания
-
private
void
textBox1_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
textBox2.Select();
-
//textBox2.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Конечные показания активирует текстовое поле Цена за 1 Квт/час
-
private
void
textBox2_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
textBox3.Select();
-
//textBox3.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Цена за 1 Квт/час активирует кнопку Расчитать
-
private
void
textBox3_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
button1.Select();
-
//button1.Focus();
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
//текстовое поле Сумма активирует кнопку Очистить
-
private
void
textBox4_KeyPress(
object
sender, KeyPressEventArgs e) {
-
if
(e.KeyChar == (
char
)Keys.Enter) {
-
button2.Select();
-
//button2.Focus();
-
//если в текстовом поле свойство ReadOnly = true, делать перехват клавиши не обязательно
-
//перехватываем нажатие клавиши, удаляется звук
-
e.Handled =
true
;
-
}
-
}
-
}
}
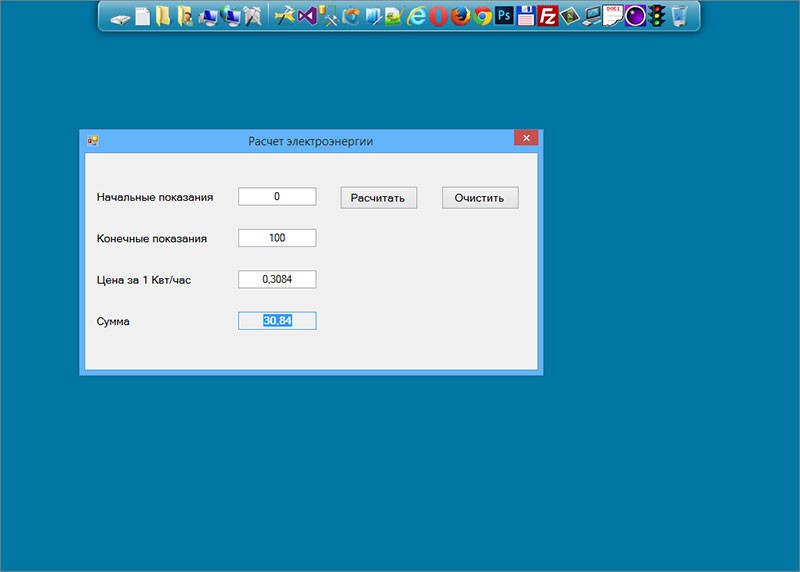