создание и заполнение таблицы программно
заполнение таблицы динамически
запись и мгновенное отображение данных
заполнение dataGridView из текстового файла
запись из dataGridView в текстовый файл
заполнение таблицы динамически
запись и мгновенное отображение данных
заполнение dataGridView из текстового файла
запись из dataGridView в текстовый файл
создание и заполнение таблицы программно
Form1.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0070 {
-
public
partial
class
Form1 : Form {
-
/*создание таблицы*/
-
DataGridView dataGridView1 =
new
DataGridView();
-
void
Funtcion() {
-
/*размер таблицы*/
-
dataGridView1.Size =
new
Size(400, 200);
-
/*создание столбцов*/
-
//1 столбец, текстовый
-
DataGridViewTextBoxColumn column0 =
new
DataGridViewTextBoxColumn();
-
column0.Name =
"id"
;
-
column0.HeaderText =
"ID"
;
-
//2 столбец, текстовый
-
DataGridViewTextBoxColumn column1 =
new
DataGridViewTextBoxColumn();
-
column1.Name =
"brand"
;
-
column1.HeaderText =
"Brand"
;
-
//3 столбец, изображение
-
DataGridViewImageColumn column2 =
new
DataGridViewImageColumn();
-
column2.Name =
"image"
;
-
column2.HeaderText =
"Image"
;
-
//добавляем столбцы
-
dataGridView1.Columns.AddRange(column0, column1, column2);
-
/*создание ячеек*/
-
//ячейки для 1 строки
-
DataGridViewCell id0 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand0 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image0 =
new
DataGridViewImageCell();
-
id0.Value =
"1"
;
-
brand0.Value =
"BMW"
;
-
image0.Value = imageList1.Images[0];
-
//создание строки
-
DataGridViewRow row0 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row0.Cells.AddRange(id0, brand0, image0);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row0);
-
//ячейки для 2 строки
-
DataGridViewCell id1 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand1 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image1 =
new
DataGridViewImageCell();
-
id1.Value =
"2"
;
-
brand1.Value =
"Bentley"
;
-
image1.Value = imageList1.Images[1];
-
//создание строки
-
DataGridViewRow row1 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row1.Cells.AddRange(id1, brand1, image1);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row1);
-
//ячейки для 3 строки
-
DataGridViewCell id2 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand2 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image2 =
new
DataGridViewImageCell();
-
id2.Value =
"3"
;
-
brand2.Value =
"Mercedes"
;
-
image2.Value = imageList1.Images[2];
-
//создание строки
-
DataGridViewRow row2 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row2.Cells.AddRange(id2, brand2, image2);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row2);
-
}
-
public
Form1() {
-
InitializeComponent();
-
//добавляем на форму таблицу
-
this
.Controls.Add(dataGridView1);
-
Funtcion();
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0070 {
-
public
partial
class
Form1 : Form {
-
/*создание таблицы*/
-
DataGridView dataGridView1 =
new
DataGridView();
-
void
Funtcion() {
-
/*размер таблицы*/
-
dataGridView1.Size =
new
Size(400, 200);
-
/*создание столбцов*/
-
//1 столбец, текстовый
-
DataGridViewTextBoxColumn column0 =
new
DataGridViewTextBoxColumn();
-
column0.Name =
"id"
;
-
column0.HeaderText =
"ID"
;
-
//2 столбец, текстовый
-
DataGridViewTextBoxColumn column1 =
new
DataGridViewTextBoxColumn();
-
column1.Name =
"brand"
;
-
column1.HeaderText =
"Brand"
;
-
//3 столбец, изображение
-
DataGridViewImageColumn column2 =
new
DataGridViewImageColumn();
-
column2.Name =
"image"
;
-
column2.HeaderText =
"Image"
;
-
//добавляем столбцы
-
dataGridView1.Columns.AddRange(column0, column1, column2);
-
/*создание ячеек*/
-
//ячейки для 1 строки
-
DataGridViewCell id0 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand0 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image0 =
new
DataGridViewImageCell();
-
id0.Value =
"1"
;
-
brand0.Value =
"BMW"
;
-
image0.Value = imageList1.Images[0];
-
//создание строки
-
DataGridViewRow row0 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row0.Cells.AddRange(id0, brand0, image0);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row0);
-
//ячейки для 2 строки
-
DataGridViewCell id1 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand1 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image1 =
new
DataGridViewImageCell();
-
id1.Value =
"2"
;
-
brand1.Value =
"Bentley"
;
-
image1.Value = imageList1.Images[1];
-
//создание строки
-
DataGridViewRow row1 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row1.Cells.AddRange(id1, brand1, image1);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row1);
-
//ячейки для 3 строки
-
DataGridViewCell id2 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell brand2 =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image2 =
new
DataGridViewImageCell();
-
id2.Value =
"3"
;
-
brand2.Value =
"Mercedes"
;
-
image2.Value = imageList1.Images[2];
-
//создание строки
-
DataGridViewRow row2 =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row2.Cells.AddRange(id2, brand2, image2);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row2);
-
}
-
public
Form1() {
-
InitializeComponent();
-
//добавляем на форму таблицу
-
this
.Controls.Add(dataGridView1);
-
Funtcion();
-
}
-
}
}
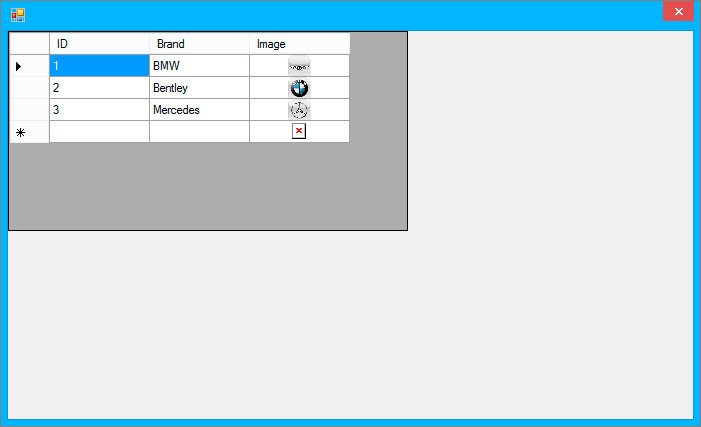
заполнение таблицы динамически
Form1.cs
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0071 {
-
public
partial
class
Form1 : Form {
-
int
counter = 0;
-
public
Form1() {
-
InitializeComponent();
-
}
-
//Browse image
-
private
void
buttonBrowse_Click(
object
sender, EventArgs e) {
-
openFileDialog1.Filter =
"Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"
;
-
if
(openFileDialog1.ShowDialog() == DialogResult.OK) {
-
imageList1.Images.Add(Image.FromFile(openFileDialog1.FileName));
-
}
-
}
-
//Add
-
private
void
buttonAdd_Click(
object
sender, EventArgs e) {
-
//создание ячеек
-
DataGridViewCell brand =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image =
new
DataGridViewImageCell();
-
//заполнение ячеек
-
brand.Value = textBoxBrend.Text;
-
image.Value = imageList1.Images[counter++];
-
//создание строки
-
DataGridViewRow row =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row.Cells.AddRange(brand, image);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row);
-
textBoxBrend.Clear();
-
//обновляем таблицу
-
dataGridView1.Refresh();
-
textBoxBrend.Select();
-
}
-
//Clear
-
private
void
buttonClear_Click(
object
sender, EventArgs e) {
-
dataGridView1.Rows.Clear();
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
using
System.Windows.Forms;
namespace
_0071 {
-
public
partial
class
Form1 : Form {
-
int
counter = 0;
-
public
Form1() {
-
InitializeComponent();
-
}
-
//Browse image
-
private
void
buttonBrowse_Click(
object
sender, EventArgs e) {
-
openFileDialog1.Filter =
"Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"
;
-
if
(openFileDialog1.ShowDialog() == DialogResult.OK) {
-
imageList1.Images.Add(Image.FromFile(openFileDialog1.FileName));
-
}
-
}
-
//Add
-
private
void
buttonAdd_Click(
object
sender, EventArgs e) {
-
//создание ячеек
-
DataGridViewCell brand =
new
DataGridViewTextBoxCell();
-
DataGridViewCell image =
new
DataGridViewImageCell();
-
//заполнение ячеек
-
brand.Value = textBoxBrend.Text;
-
image.Value = imageList1.Images[counter++];
-
//создание строки
-
DataGridViewRow row =
new
DataGridViewRow();
-
//добавление ячеек в строку
-
row.Cells.AddRange(brand, image);
-
//добавление строки в таблицу
-
dataGridView1.Rows.Add(row);
-
textBoxBrend.Clear();
-
//обновляем таблицу
-
dataGridView1.Refresh();
-
textBoxBrend.Select();
-
}
-
//Clear
-
private
void
buttonClear_Click(
object
sender, EventArgs e) {
-
dataGridView1.Rows.Clear();
-
}
-
}
}
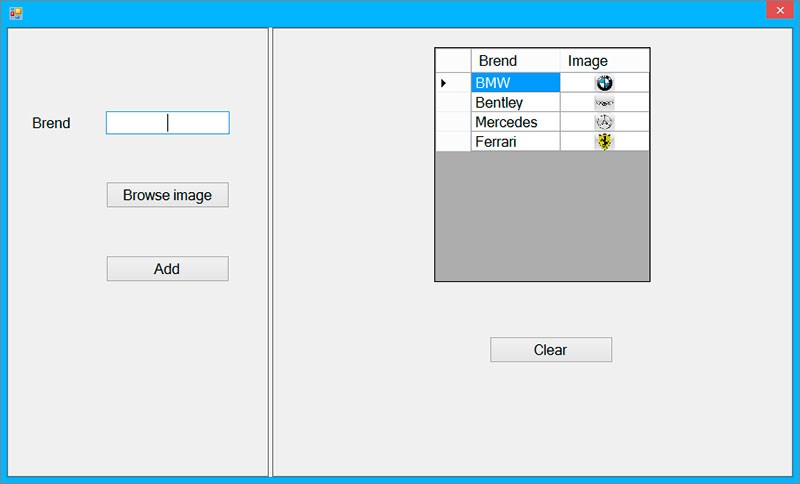
запись и мгновенное отображение данных
Создайте базу данных "abc" и таблицу "Avto".
*.sql
CREATE DATABASE abc GO USE abc GO CREATE TABLE [dbo].[Avto] ( [Id] INT IDENTITY (1, 1) NOT NULL, [Brand] VARCHAR (255) NOT NULL, [ImageBrand] VARBINARY (MAX) NOT NULL, [Country] VARCHAR (255) NOT NULL, [ImageCountry] IMAGE NOT NULL, CONSTRAINT [PK_Avto] PRIMARY KEY CLUSTERED ([Id] ASC) );
CREATE DATABASE abc GO USE abc GO CREATE TABLE [dbo].[Avto] ( [Id] INT IDENTITY (1, 1) NOT NULL, [Brand] VARCHAR (255) NOT NULL, [ImageBrand] VARBINARY (MAX) NOT NULL, [Country] VARCHAR (255) NOT NULL, [ImageCountry] IMAGE NOT NULL, CONSTRAINT [PK_Avto] PRIMARY KEY CLUSTERED ([Id] ASC) );
Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; using System.IO; namespace _0072 { public partial class Form1 : Form { string pathImageBrand = string.Empty; string pathImageCountry = string.Empty; public Form1() { InitializeComponent(); } //кнопка Brand / Browse private void buttonBrowseBrand_Click(object sender, EventArgs e) { openFileDialog1.Filter = "Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"; if(openFileDialog1.ShowDialog() == DialogResult.OK) { pathImageBrand = openFileDialog1.FileName.ToString(); } } //кнопка Country / Browse private void buttonBrowseCountry_Click(object sender, EventArgs e) { openFileDialog2.Filter = "Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"; if(openFileDialog2.ShowDialog() == DialogResult.OK) { pathImageCountry = openFileDialog2.FileName.ToString(); } } //кнопка Add private void buttonAdd_Click(object sender, EventArgs e) { string stringConnect = @"Data Source=BISEM\MSSQLSERVER2012;Initial Catalog=abc;Integrated Security=True"; string sql = "INSERT INTO Avto VALUES("+null+" '"+textBoxBrand.Text+"', @imgBrands, '"+textBoxCountry.Text+"', @imgCountries)"; byte[] imgBrands = null; FileStream fsBrand = new FileStream(pathImageBrand, FileMode.Open, FileAccess.Read); BinaryReader brBrand = new BinaryReader(fsBrand); imgBrands = brBrand.ReadBytes((int)fsBrand.Length); byte[] imgCountries = null; FileStream fsCountry = new FileStream(pathImageCountry, FileMode.Open, FileAccess.Read); BinaryReader brCountry = new BinaryReader(fsCountry); imgCountries = brCountry.ReadBytes((int)fsCountry.Length); using(SqlConnection connect = new SqlConnection(stringConnect)) { try { connect.Open(); SqlCommand command = new SqlCommand(sql, connect); command.Parameters.Add(new SqlParameter("@imgBrands", imgBrands)); command.Parameters.Add(new SqlParameter("@imgCountries", imgCountries)); int x = command.ExecuteNonQuery(); MessageBox.Show(x.ToString() + " Record added"); } catch(Exception exc) { MessageBox.Show("Error connection with a database {0}", exc.Message); } } textBoxBrand.Clear(); textBoxCountry.Clear(); this.avtoTableAdapter.Fill(this.abcDataSet.Avto); textBoxBrand.Select(); } private void Form1_Load(object sender, EventArgs e) { // TODO: This line of code loads data into the 'abcDataSet.Avto' table. You can move, or remove it, as needed. this.avtoTableAdapter.Fill(this.abcDataSet.Avto); } } }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; using System.IO; namespace _0072 { public partial class Form1 : Form { string pathImageBrand = string.Empty; string pathImageCountry = string.Empty; public Form1() { InitializeComponent(); } //кнопка Brand / Browse private void buttonBrowseBrand_Click(object sender, EventArgs e) { openFileDialog1.Filter = "Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"; if(openFileDialog1.ShowDialog() == DialogResult.OK) { pathImageBrand = openFileDialog1.FileName.ToString(); } } //кнопка Country / Browse private void buttonBrowseCountry_Click(object sender, EventArgs e) { openFileDialog2.Filter = "Images (*.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png) | *.jpg; *.jpeg; *.gif; *.bmp; *.ico; *.png"; if(openFileDialog2.ShowDialog() == DialogResult.OK) { pathImageCountry = openFileDialog2.FileName.ToString(); } } //кнопка Add private void buttonAdd_Click(object sender, EventArgs e) { string stringConnect = @"Data Source=BISEM\MSSQLSERVER2012;Initial Catalog=abc;Integrated Security=True"; string sql = "INSERT INTO Avto VALUES("+null+" '"+textBoxBrand.Text+"', @imgBrands, '"+textBoxCountry.Text+"', @imgCountries)"; byte[] imgBrands = null; FileStream fsBrand = new FileStream(pathImageBrand, FileMode.Open, FileAccess.Read); BinaryReader brBrand = new BinaryReader(fsBrand); imgBrands = brBrand.ReadBytes((int)fsBrand.Length); byte[] imgCountries = null; FileStream fsCountry = new FileStream(pathImageCountry, FileMode.Open, FileAccess.Read); BinaryReader brCountry = new BinaryReader(fsCountry); imgCountries = brCountry.ReadBytes((int)fsCountry.Length); using(SqlConnection connect = new SqlConnection(stringConnect)) { try { connect.Open(); SqlCommand command = new SqlCommand(sql, connect); command.Parameters.Add(new SqlParameter("@imgBrands", imgBrands)); command.Parameters.Add(new SqlParameter("@imgCountries", imgCountries)); int x = command.ExecuteNonQuery(); MessageBox.Show(x.ToString() + " Record added"); } catch(Exception exc) { MessageBox.Show("Error connection with a database {0}", exc.Message); } } textBoxBrand.Clear(); textBoxCountry.Clear(); this.avtoTableAdapter.Fill(this.abcDataSet.Avto); textBoxBrand.Select(); } private void Form1_Load(object sender, EventArgs e) { // TODO: This line of code loads data into the 'abcDataSet.Avto' table. You can move, or remove it, as needed. this.avtoTableAdapter.Fill(this.abcDataSet.Avto); } } }
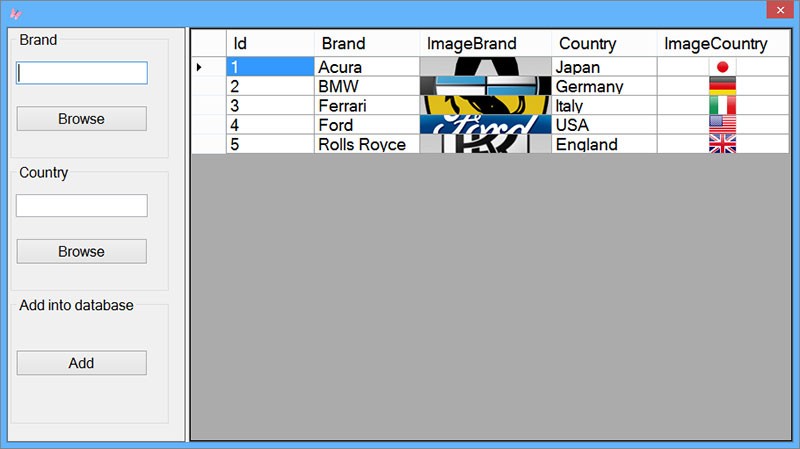
База данных находится в папке "database" в архиве.
заполнение dataGridView из текстового файла
Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; namespace _0073 { public partial class Form1 : Form { //метод заполняет dataGridView данными из текстового файла public void Function() { DataSet ds = new DataSet(); //создаем временную таблицу ds.Tables.Add("Temp"); //путь к текстовому файлу string path = @"a.txt"; StreamReader sr = new StreamReader(path); /*создаем колонки в таблице и заполняем их названиями*/ //считываем первую строку из файла, в ней названия столбцов string firstLine = sr.ReadLine(); //массив имен колонок из файла string[] arraNameColumn = System.Text.RegularExpressions.Regex.Split(firstLine, ","); for(int i=0; i<arraNameColumn.Length; i++) { ds.Tables[0].Columns.Add(arraNameColumn[i]); } /*заполняем строки в таблице*/ string Line = sr.ReadLine(); while(Line != null) { string[] arraCell = System.Text.RegularExpressions.Regex.Split(Line, ","); ds.Tables[0].Rows.Add(arraCell); Line = sr.ReadLine(); } //привязываем dataGridView к таблице dataGridView1.DataSource = ds.Tables[0]; } public Form1() { InitializeComponent(); Function(); } } }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; namespace _0073 { public partial class Form1 : Form { //метод заполняет dataGridView данными из текстового файла public void Function() { DataSet ds = new DataSet(); //создаем временную таблицу ds.Tables.Add("Temp"); //путь к текстовому файлу string path = @"a.txt"; StreamReader sr = new StreamReader(path); /*создаем колонки в таблице и заполняем их названиями*/ //считываем первую строку из файла, в ней названия столбцов string firstLine = sr.ReadLine(); //массив имен колонок из файла string[] arraNameColumn = System.Text.RegularExpressions.Regex.Split(firstLine, ","); for(int i=0; i<arraNameColumn.Length; i++) { ds.Tables[0].Columns.Add(arraNameColumn[i]); } /*заполняем строки в таблице*/ string Line = sr.ReadLine(); while(Line != null) { string[] arraCell = System.Text.RegularExpressions.Regex.Split(Line, ","); ds.Tables[0].Rows.Add(arraCell); Line = sr.ReadLine(); } //привязываем dataGridView к таблице dataGridView1.DataSource = ds.Tables[0]; } public Form1() { InitializeComponent(); Function(); } } }
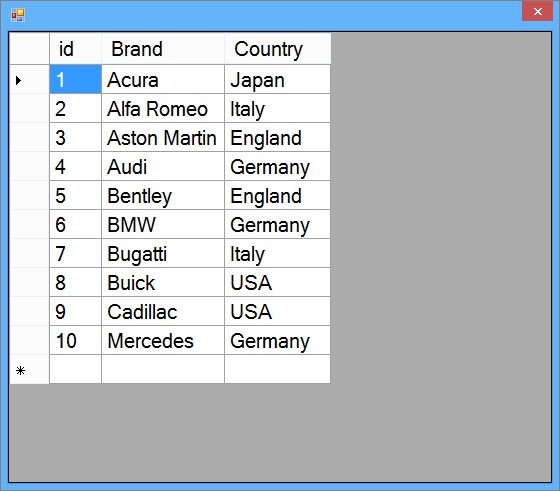
запись из dataGridView в текстовый файл
Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; namespace _0074 { public partial class Form1 : Form { string path = string.Empty; public Form1() { InitializeComponent(); } //кнопка Browse file private void button1_Click(object sender, EventArgs e) { openFileDialog1.Filter = "Text (*.txt) | *.txt"; if(openFileDialog1.ShowDialog() == DialogResult.OK) { path = openFileDialog1.FileName; } } //кнопка Record into file private void button2_Click(object sender, EventArgs e) { using(StreamWriter sw = new StreamWriter(path, true)) { for(int i = 0; i < Convert.ToInt32(dataGridView1.Rows.Count-1); i++) { sw.WriteLine(dataGridView1.Rows[i].Cells[0].Value.ToString() + "\t" + dataGridView1.Rows[i].Cells[1].Value.ToString()); } } } } }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; namespace _0074 { public partial class Form1 : Form { string path = string.Empty; public Form1() { InitializeComponent(); } //кнопка Browse file private void button1_Click(object sender, EventArgs e) { openFileDialog1.Filter = "Text (*.txt) | *.txt"; if(openFileDialog1.ShowDialog() == DialogResult.OK) { path = openFileDialog1.FileName; } } //кнопка Record into file private void button2_Click(object sender, EventArgs e) { using(StreamWriter sw = new StreamWriter(path, true)) { for(int i = 0; i < Convert.ToInt32(dataGridView1.Rows.Count-1); i++) { sw.WriteLine(dataGridView1.Rows[i].Cells[0].Value.ToString() + "\t" + dataGridView1.Rows[i].Cells[1].Value.ToString()); } } } } }
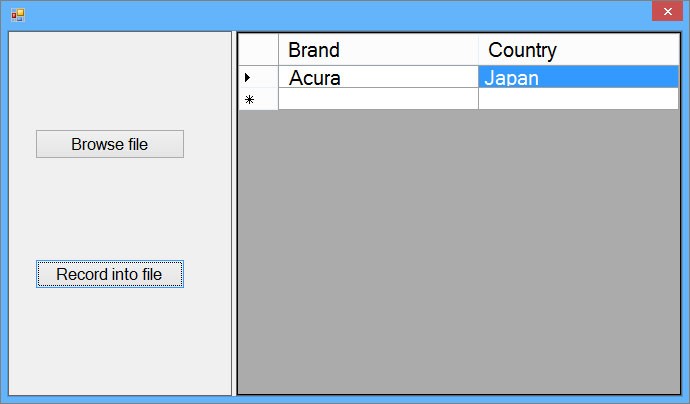