Default.aspx
<%@ Page Language="C#" %> <!DOCTYPE html> <%@ Import Namespace="Style_Of_ASP" %> <script runat="server"> </script> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title>Расчет электроенергии</title> <link href="css/style.css" rel="stylesheet" /> </head> <body> <form id="form1" runat="server"> <% string showStart = null; string showEnd = null; string price = null; if(Request.HttpMethod == "POST") { //делаем view state if(Request["calculate"] != null) { showStart = Request["showStart"]; showEnd = Request["showEnd"]; price = Request["price"]; } if(Request["clear"] != null) { showStart = null; showEnd = null; price = null; } } %> <div class="a"> <input type="text" name="showStart" value="<% Response.Write(showStart); %>" /> <label>Начальные показания</label> <br /> <br /> <input type="text" name="showEnd" value="<% Response.Write(showEnd); %>" /> <label>Конечные показания</label> <br /> <br /> <input type="text" name="price" value="<% Response.Write(price); %>" /> <label>Цена за 1кВт/ч</label> <br /> <br /> <input id="calculate" type="submit" name="calculate" value="Расчитать" /> <br /> <br /> <input id="clear" type="submit" name="clear" value="Очистить" /> <div id="b" class="b"> <label id="result"> <% if(Request.HttpMethod == "POST" && Request["calculate"] != null && Request["clear"] == null) { Counter C = new Counter(showStart, showEnd, price); Response.Write("Сумма = " + C.Sum().ToString()); } %> </label> </div> </div> </form> </body> </html>
<%@ Page Language="C#" %> <!DOCTYPE html> <%@ Import Namespace="Style_Of_ASP" %> <script runat="server"> </script> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title>Расчет электроенергии</title> <link href="css/style.css" rel="stylesheet" /> </head> <body> <form id="form1" runat="server"> <% string showStart = null; string showEnd = null; string price = null; if(Request.HttpMethod == "POST") { //делаем view state if(Request["calculate"] != null) { showStart = Request["showStart"]; showEnd = Request["showEnd"]; price = Request["price"]; } if(Request["clear"] != null) { showStart = null; showEnd = null; price = null; } } %> <div class="a"> <input type="text" name="showStart" value="<% Response.Write(showStart); %>" /> <label>Начальные показания</label> <br /> <br /> <input type="text" name="showEnd" value="<% Response.Write(showEnd); %>" /> <label>Конечные показания</label> <br /> <br /> <input type="text" name="price" value="<% Response.Write(price); %>" /> <label>Цена за 1кВт/ч</label> <br /> <br /> <input id="calculate" type="submit" name="calculate" value="Расчитать" /> <br /> <br /> <input id="clear" type="submit" name="clear" value="Очистить" /> <div id="b" class="b"> <label id="result"> <% if(Request.HttpMethod == "POST" && Request["calculate"] != null && Request["clear"] == null) { Counter C = new Counter(showStart, showEnd, price); Response.Write("Сумма = " + C.Sum().ToString()); } %> </label> </div> </div> </form> </body> </html>
css/style.css
* { margin: 0; padding: 0; } .a { height: 298px; width: 448px; position: absolute; top: 50%; left: 50%; margin-top: -175px; margin-left: -250px; padding: 25px; background-color: #D3E0ED; border: 1px solid #FFB300; border-radius: 20px; } input, label { font-size: 20px; text-align: center; width: 200px; } #calculate, #clear { cursor: pointer; } .b { height: 60px; width: 400px; margin: 25px 25px 25px 25px; line-height: 60px; text-align: center; font-size: 22px; border: 1px solid #FFB300; border-radius: 10px; } .b label { font-size: 24px; }
* { margin: 0; padding: 0; } .a { height: 298px; width: 448px; position: absolute; top: 50%; left: 50%; margin-top: -175px; margin-left: -250px; padding: 25px; background-color: #D3E0ED; border: 1px solid #FFB300; border-radius: 20px; } input, label { font-size: 20px; text-align: center; width: 200px; } #calculate, #clear { cursor: pointer; } .b { height: 60px; width: 400px; margin: 25px 25px 25px 25px; line-height: 60px; text-align: center; font-size: 22px; border: 1px solid #FFB300; border-radius: 10px; } .b label { font-size: 24px; }
App_Code/Counter.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace Style_Of_ASP { public class Counter { ushort showStart; ushort showEnd; decimal price; public Counter(string arg0, string arg1, string arg2) { ushort.TryParse(arg0, out showStart); ushort.TryParse(arg1, out showEnd); decimal.TryParse(arg2, out price); } public ushort ResultShow() { return (ushort)(showEnd - showStart); } public decimal Sum() { return Math.Round((decimal)ResultShow()*price, 2); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace Style_Of_ASP { public class Counter { ushort showStart; ushort showEnd; decimal price; public Counter(string arg0, string arg1, string arg2) { ushort.TryParse(arg0, out showStart); ushort.TryParse(arg1, out showEnd); decimal.TryParse(arg2, out price); } public ushort ResultShow() { return (ushort)(showEnd - showStart); } public decimal Sum() { return Math.Round((decimal)ResultShow()*price, 2); } } }
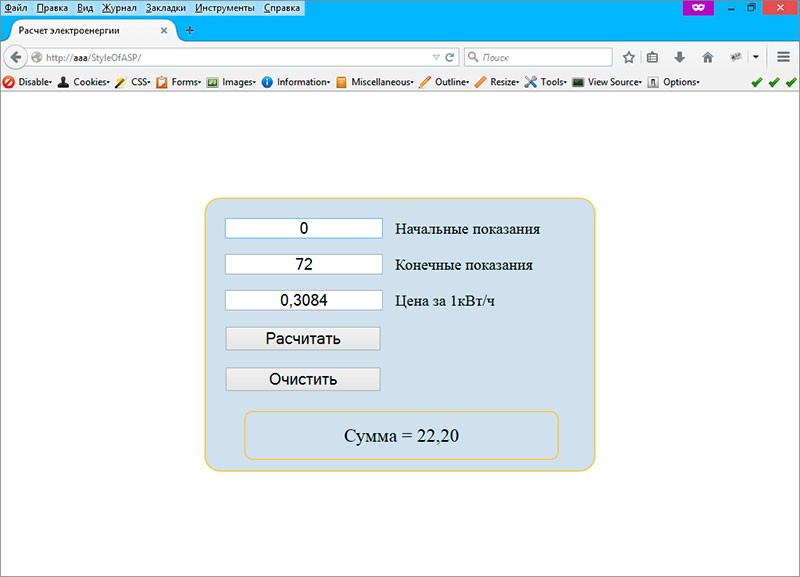