При возникновении любой ошибки, желтая страница смерти не будет доступна для пользователя, а произойдет перенаправление на специально созданную страницу. После определенного времени произойдет перенаправление на главную страницу.
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox> <asp:DropDownList ID="DropDownList1" runat="server"> <asp:ListItem>+</asp:ListItem> <asp:ListItem>-</asp:ListItem> <asp:ListItem>*</asp:ListItem> <asp:ListItem>/</asp:ListItem> <asp:ListItem>%</asp:ListItem> </asp:DropDownList> <asp:TextBox ID="TextBox2" runat="server"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="=" OnClick="Button1_Click" /> <asp:TextBox ID="TextBox3" runat="server"></asp:TextBox> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox> <asp:DropDownList ID="DropDownList1" runat="server"> <asp:ListItem>+</asp:ListItem> <asp:ListItem>-</asp:ListItem> <asp:ListItem>*</asp:ListItem> <asp:ListItem>/</asp:ListItem> <asp:ListItem>%</asp:ListItem> </asp:DropDownList> <asp:TextBox ID="TextBox2" runat="server"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="=" OnClick="Button1_Click" /> <asp:TextBox ID="TextBox3" runat="server"></asp:TextBox> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { if((sender as Button).Text == "=") { (sender as Button).Text = "Clear"; decimal a = decimal.Parse(TextBox1.Text); decimal b = decimal.Parse(TextBox2.Text); switch(DropDownList1.Text) { case "+": TextBox3.Text = ((decimal)(a + b)).ToString(); break; case "-": TextBox3.Text = ((decimal)(a - b)).ToString(); break; case "*": TextBox3.Text = ((decimal)(a * b)).ToString(); break; case "/": if(b == 0) { throw new Exception("На ноль делить нельзя!"); } else { TextBox3.Text = ((decimal)(a / b)).ToString(); } break; case "%": if(b == 0) { throw new Exception("На ноль делить нельзя!"); } else { TextBox3.Text = ((decimal)(a % b)).ToString(); } break; } } else { (sender as Button).Text = "="; TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; DropDownList1.Text = "+"; } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { if((sender as Button).Text == "=") { (sender as Button).Text = "Clear"; decimal a = decimal.Parse(TextBox1.Text); decimal b = decimal.Parse(TextBox2.Text); switch(DropDownList1.Text) { case "+": TextBox3.Text = ((decimal)(a + b)).ToString(); break; case "-": TextBox3.Text = ((decimal)(a - b)).ToString(); break; case "*": TextBox3.Text = ((decimal)(a * b)).ToString(); break; case "/": if(b == 0) { throw new Exception("На ноль делить нельзя!"); } else { TextBox3.Text = ((decimal)(a / b)).ToString(); } break; case "%": if(b == 0) { throw new Exception("На ноль делить нельзя!"); } else { TextBox3.Text = ((decimal)(a % b)).ToString(); } break; } } else { (sender as Button).Text = "="; TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; DropDownList1.Text = "+"; } } }
error.html
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <title></title> <style> body { background-color: #f5fbff; } .main { height: 300px; width: 300px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -150px; background-color: #FFF; border-radius: 15px; } .a { height: 225px; width: 300px; } img { height: 225px; width: 225px; position: relative; top: 50%; left: 50%; margin-top: -112.5px; margin-left: -112.5px; } .b { height: 75px; width: 300px; line-height: 75px; text-align: center; color: #00F; font-size: 18px; } </style> </head> <body> <div class="main"> <div class="a"> <img src="img/error.png" /> </div> <div class="b"> На главную страницу через <span id="s1">5</span> </div> </div> <script> var i = 5; function funMessage() { i--; if(i == -1) { location.replace('Default.aspx'); } else { document.getElementById('s1').innerHTML = i; } } function funMake() { setInterval('funMessage()', 1000); } window.onload = function () { funMake(); } </script> </body> </html>
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <title></title> <style> body { background-color: #f5fbff; } .main { height: 300px; width: 300px; position: absolute; top: 50%; left: 50%; margin-top: -150px; margin-left: -150px; background-color: #FFF; border-radius: 15px; } .a { height: 225px; width: 300px; } img { height: 225px; width: 225px; position: relative; top: 50%; left: 50%; margin-top: -112.5px; margin-left: -112.5px; } .b { height: 75px; width: 300px; line-height: 75px; text-align: center; color: #00F; font-size: 18px; } </style> </head> <body> <div class="main"> <div class="a"> <img src="img/error.png" /> </div> <div class="b"> На главную страницу через <span id="s1">5</span> </div> </div> <script> var i = 5; function funMessage() { i--; if(i == -1) { location.replace('Default.aspx'); } else { document.getElementById('s1').innerHTML = i; } } function funMake() { setInterval('funMessage()', 1000); } window.onload = function () { funMake(); } </script> </body> </html>
Web.config
<?xml version="1.0"?> <configuration> <system.web> <compilation debug="true" targetFramework="4.5" /> <httpRuntime targetFramework="4.5" /> <!--RemoteOnly мы видим, а пользователи с других пк не видят желтую страницу с ошибками--> <!--On мы и пользователи с других пк не видят желтую страницу с ошибками--> <customErrors mode="On"> <!--страница не найдена--> <error statusCode="404" redirect="~/error.html"/> <!--500 - ошибка на сервере--> <error statusCode="500" redirect="~/error.html"/> </customErrors> </system.web> </configuration>
<?xml version="1.0"?> <configuration> <system.web> <compilation debug="true" targetFramework="4.5" /> <httpRuntime targetFramework="4.5" /> <!--RemoteOnly мы видим, а пользователи с других пк не видят желтую страницу с ошибками--> <!--On мы и пользователи с других пк не видят желтую страницу с ошибками--> <customErrors mode="On"> <!--страница не найдена--> <error statusCode="404" redirect="~/error.html"/> <!--500 - ошибка на сервере--> <error statusCode="500" redirect="~/error.html"/> </customErrors> </system.web> </configuration>
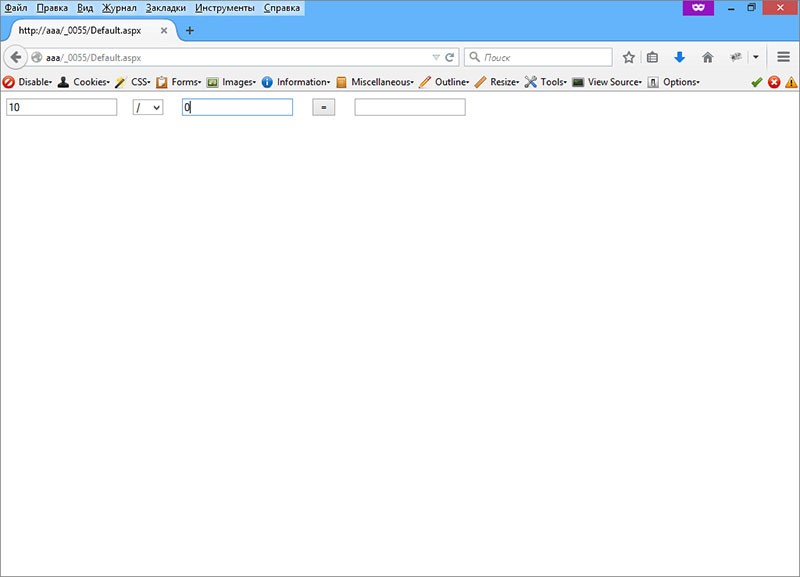
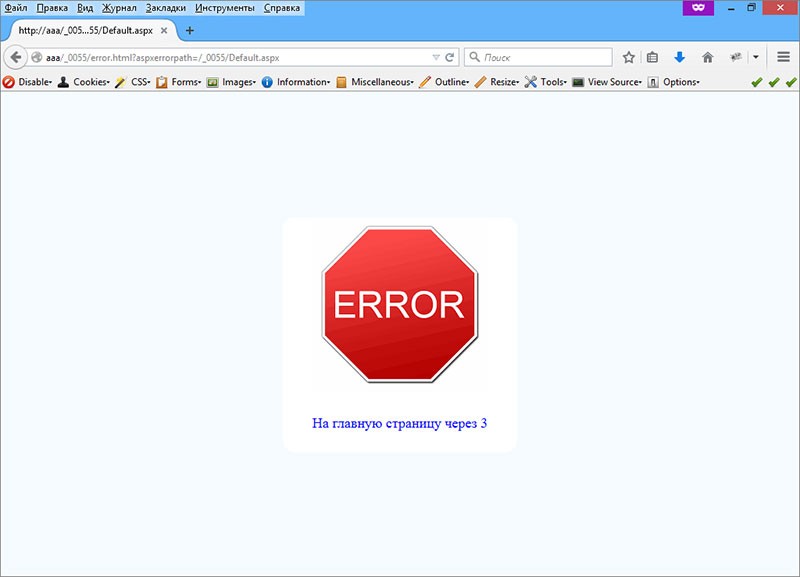