CAPTCHA -Completely Automated Public Turing test to tell Computers and Humans Apart —полностью автоматизированный публичный тест Тьюринга для различения компьютеров и людей.
Captcha.ashx
<%@ WebHandler Language="C#" Class="Captcha" %> using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Drawing; using System.Drawing.Imaging; using System.Security.Cryptography; using System.Text; using System.Web.SessionState; public class Captcha : IHttpHandler, IRequiresSessionState { Random rand = new Random(); public void ProcessRequest(HttpContext context) { /*указываем тип ответа данного хэндлера*/ context.Response.ContentType = "image/jpeg"; /*высота и ширина картинки*/ int height = 60; int width = 100; /*объект изображения*/ Bitmap captchaImage = new Bitmap(width, height); /*объект для изменения пикелей изображения*/ Graphics gr = Graphics.FromImage(captchaImage); /*создание строки для вывода на изображении*/ string captchaString = GenerateCaptchaString(); /*сохраняем текст, который будет выведен на изображении в Session*/ HttpContext.Current.Session["captcha"] = captchaString; /*заполнение изображения цветом*/ gr.FillRectangle(GenerateBrush(), new Rectangle(0, 0, width, height)); /*создание кисти для рисования текста*/ Brush textBrush = GenerateBrush(); /*добавление в содержимое страницы текста*/ gr.DrawString(captchaString, new Font("Arial", 10.0f), textBrush, new Point(20, 20)); /*сохранение изображения в ответ пользователю*/ captchaImage.Save(context.Response.OutputStream, ImageFormat.Jpeg); /*завершение формирования ответа и отправка изображения пользователю*/ context.Response.End(); } /*метод для создания текста*/ private string GenerateCaptchaString() { string symbols = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; string captchaString = string.Empty; for(int i = 0; i < 7; i++) { int pos = rand.Next(0, symbols.Length); captchaString += symbols[pos]; } return captchaString; } /*метод для создания случайного цвета*/ private Brush GenerateBrush() { int r = rand.Next(255); int g = rand.Next(255); int b = rand.Next(255); return new SolidBrush(Color.FromArgb(r, g, b)); } public bool IsReusable { get { return false; } } }
<%@ WebHandler Language="C#" Class="Captcha" %> using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Drawing; using System.Drawing.Imaging; using System.Security.Cryptography; using System.Text; using System.Web.SessionState; public class Captcha : IHttpHandler, IRequiresSessionState { Random rand = new Random(); public void ProcessRequest(HttpContext context) { /*указываем тип ответа данного хэндлера*/ context.Response.ContentType = "image/jpeg"; /*высота и ширина картинки*/ int height = 60; int width = 100; /*объект изображения*/ Bitmap captchaImage = new Bitmap(width, height); /*объект для изменения пикелей изображения*/ Graphics gr = Graphics.FromImage(captchaImage); /*создание строки для вывода на изображении*/ string captchaString = GenerateCaptchaString(); /*сохраняем текст, который будет выведен на изображении в Session*/ HttpContext.Current.Session["captcha"] = captchaString; /*заполнение изображения цветом*/ gr.FillRectangle(GenerateBrush(), new Rectangle(0, 0, width, height)); /*создание кисти для рисования текста*/ Brush textBrush = GenerateBrush(); /*добавление в содержимое страницы текста*/ gr.DrawString(captchaString, new Font("Arial", 10.0f), textBrush, new Point(20, 20)); /*сохранение изображения в ответ пользователю*/ captchaImage.Save(context.Response.OutputStream, ImageFormat.Jpeg); /*завершение формирования ответа и отправка изображения пользователю*/ context.Response.End(); } /*метод для создания текста*/ private string GenerateCaptchaString() { string symbols = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; string captchaString = string.Empty; for(int i = 0; i < 7; i++) { int pos = rand.Next(0, symbols.Length); captchaString += symbols[pos]; } return captchaString; } /*метод для создания случайного цвета*/ private Brush GenerateBrush() { int r = rand.Next(255); int g = rand.Next(255); int b = rand.Next(255); return new SolidBrush(Color.FromArgb(r, g, b)); } public bool IsReusable { get { return false; } } }
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> .red { color: #FF0000; } td { padding: 5px; } #Button1, #Button2 { cursor: pointer; } </style> </head> <body> <form id="form1" runat="server"> <div> <table> <tr> <td><img src="Captcha.ashx" /></td> <td> <asp:Button ID="Button2" runat="server" Text="Обновить картинку" OnClick="Button2_Click" /> </td> <td>введите текст</td> <td> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox> </td> <td> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> </td> <td> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </td> </tr> </table> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> .red { color: #FF0000; } td { padding: 5px; } #Button1, #Button2 { cursor: pointer; } </style> </head> <body> <form id="form1" runat="server"> <div> <table> <tr> <td><img src="Captcha.ashx" /></td> <td> <asp:Button ID="Button2" runat="server" Text="Обновить картинку" OnClick="Button2_Click" /> </td> <td>введите текст</td> <td> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox> </td> <td> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> </td> <td> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </td> </tr> </table> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { string captcha = Session["captcha"] as string; string str = TextBox1.Text.ToUpper(); if(captcha == str) { Label1.Text = "Yes!"; } else { Label1.CssClass = "red"; Label1.Text = "Error!"; } } protected void Button2_Click(object sender, EventArgs e) { } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { string captcha = Session["captcha"] as string; string str = TextBox1.Text.ToUpper(); if(captcha == str) { Label1.Text = "Yes!"; } else { Label1.CssClass = "red"; Label1.Text = "Error!"; } } protected void Button2_Click(object sender, EventArgs e) { } }
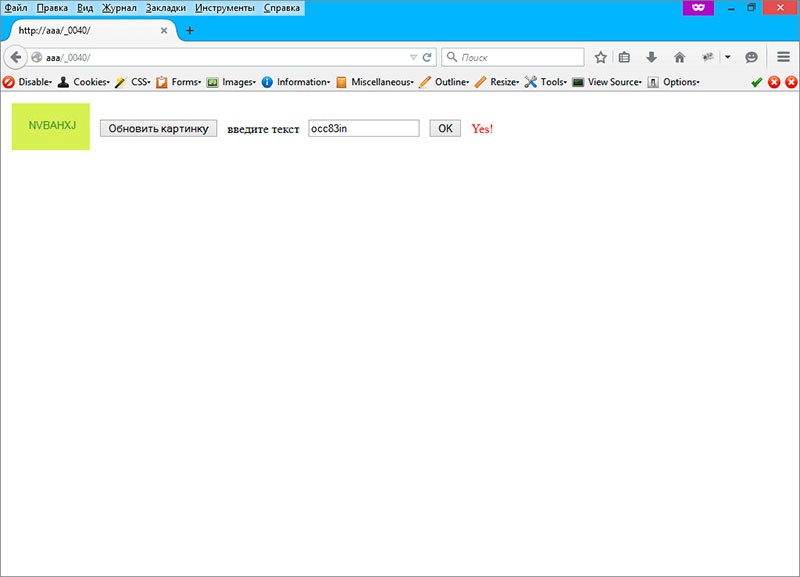