Web.config
<?xml version="1.0"?> <configuration> <system.web> <compilation debug="true" targetFramework="4.5" /> <httpRuntime targetFramework="4.5" /> </system.web> <!--информация для подключения к серверу и отправки сообщения--> <appSettings> <add key="server" value="smtp.gmail.com"/> <add key="port" value="587"/> <add key="login" value="abc@gmail.com"/> <add key="password" value="12345"/> </appSettings> </configuration>
<?xml version="1.0"?> <configuration> <system.web> <compilation debug="true" targetFramework="4.5" /> <httpRuntime targetFramework="4.5" /> </system.web> <!--информация для подключения к серверу и отправки сообщения--> <appSettings> <add key="server" value="smtp.gmail.com"/> <add key="port" value="587"/> <add key="login" value="abc@gmail.com"/> <add key="password" value="12345"/> </appSettings> </configuration>
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> td { padding-bottom: 10px; } .blue { color: #0000FF; } .red { color: #FF0000; } #Button1, #Button2 { cursor: pointer; } </style> </head> <body> <form id="form1" runat="server"> <table> <tr> <td> Кому </td> <td> <asp:TextBox ID="TextBox1" runat="server" Width="181px"></asp:TextBox> <asp:DropDownList ID="DropDownList1" runat="server"> <asp:ListItem></asp:ListItem> <asp:ListItem>@gmail.com</asp:ListItem> <asp:ListItem>@ukr.net</asp:ListItem> <asp:ListItem>@mail.ru</asp:ListItem> <asp:ListItem>@inbox.ru</asp:ListItem> <asp:ListItem>@list.ru</asp:ListItem> <asp:ListItem>@bk.ru</asp:ListItem> <asp:ListItem>@mail.ua</asp:ListItem> <asp:ListItem>@yahoo.com</asp:ListItem> <asp:ListItem>@yandex.ru</asp:ListItem> </asp:DropDownList> </td> </tr> <tr> <td> Тема </td> <td> <asp:TextBox ID="TextBox2" runat="server" Width="181px"></asp:TextBox> </td> </tr> <tr> <td> Сообщение </td> <td> <asp:TextBox ID="TextBox3" runat="server" TextMode="MultiLine" Width="181px"></asp:TextBox> </td> </tr> <tr> <td> <asp:Button ID="Button1" runat="server" Text="Отправить" OnClick="Button1_Click" /> </td> <td></td> </tr> <tr> <td> <asp:Button ID="Button2" runat="server" Text="Очистить" OnClick="Button2_Click" Width="93px" /> </td> <td></td> </tr> </table> <br /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> td { padding-bottom: 10px; } .blue { color: #0000FF; } .red { color: #FF0000; } #Button1, #Button2 { cursor: pointer; } </style> </head> <body> <form id="form1" runat="server"> <table> <tr> <td> Кому </td> <td> <asp:TextBox ID="TextBox1" runat="server" Width="181px"></asp:TextBox> <asp:DropDownList ID="DropDownList1" runat="server"> <asp:ListItem></asp:ListItem> <asp:ListItem>@gmail.com</asp:ListItem> <asp:ListItem>@ukr.net</asp:ListItem> <asp:ListItem>@mail.ru</asp:ListItem> <asp:ListItem>@inbox.ru</asp:ListItem> <asp:ListItem>@list.ru</asp:ListItem> <asp:ListItem>@bk.ru</asp:ListItem> <asp:ListItem>@mail.ua</asp:ListItem> <asp:ListItem>@yahoo.com</asp:ListItem> <asp:ListItem>@yandex.ru</asp:ListItem> </asp:DropDownList> </td> </tr> <tr> <td> Тема </td> <td> <asp:TextBox ID="TextBox2" runat="server" Width="181px"></asp:TextBox> </td> </tr> <tr> <td> Сообщение </td> <td> <asp:TextBox ID="TextBox3" runat="server" TextMode="MultiLine" Width="181px"></asp:TextBox> </td> </tr> <tr> <td> <asp:Button ID="Button1" runat="server" Text="Отправить" OnClick="Button1_Click" /> </td> <td></td> </tr> <tr> <td> <asp:Button ID="Button2" runat="server" Text="Очистить" OnClick="Button2_Click" Width="93px" /> </td> <td></td> </tr> </table> <br /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </form> </body> </html>
Default.aspx
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; //добавить using System.Configuration; using System.Net.Mail; using System.Net; using System.Text; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { /*---получение данных из файла web.config---*/ string server = ConfigurationManager.AppSettings["server"]; string port = ConfigurationManager.AppSettings["port"]; string login = ConfigurationManager.AppSettings["login"]; string password = ConfigurationManager.AppSettings["password"]; string email = TextBox1.Text + DropDownList1.Text; /*---настройка объекта сообщения---*/ MailMessage message = new MailMessage(); //логин из web.config message.From = new MailAddress(login); //кому message.To.Add(new MailAddress(email)); //тема message.Subject = TextBox2.Text; //сообщение message.Body = TextBox3.Text; //этот параметр допускает формат HTML message.IsBodyHtml = true; //кодировка message.BodyEncoding = Encoding.UTF8; /*---настройка клиента для подключения к серверу---*/ SmtpClient client = new SmtpClient(); //расположение сервера client.Host = server; //указываем порт Google, он отличается от стандартного 25 client.Port = Convert.ToInt32(port); //Google требует ssl client.EnableSsl = true; //авторизация client.Credentials = new NetworkCredential(login, password); /*---отправка сообщения---*/ try { client.Send(message); Label1.CssClass = "blue"; Label1.Text = "Сообщение отправлено успешно!"; } catch(Exception ex) { Label1.CssClass = "red"; Label1.Text = "Ошибка при отправке сообщения:<br />" + ex.Message; } } protected void Button2_Click(object sender, EventArgs e) { DropDownList1.ClearSelection(); TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; Label1.Text = string.Empty; } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; //добавить using System.Configuration; using System.Net.Mail; using System.Net; using System.Text; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { /*---получение данных из файла web.config---*/ string server = ConfigurationManager.AppSettings["server"]; string port = ConfigurationManager.AppSettings["port"]; string login = ConfigurationManager.AppSettings["login"]; string password = ConfigurationManager.AppSettings["password"]; string email = TextBox1.Text + DropDownList1.Text; /*---настройка объекта сообщения---*/ MailMessage message = new MailMessage(); //логин из web.config message.From = new MailAddress(login); //кому message.To.Add(new MailAddress(email)); //тема message.Subject = TextBox2.Text; //сообщение message.Body = TextBox3.Text; //этот параметр допускает формат HTML message.IsBodyHtml = true; //кодировка message.BodyEncoding = Encoding.UTF8; /*---настройка клиента для подключения к серверу---*/ SmtpClient client = new SmtpClient(); //расположение сервера client.Host = server; //указываем порт Google, он отличается от стандартного 25 client.Port = Convert.ToInt32(port); //Google требует ssl client.EnableSsl = true; //авторизация client.Credentials = new NetworkCredential(login, password); /*---отправка сообщения---*/ try { client.Send(message); Label1.CssClass = "blue"; Label1.Text = "Сообщение отправлено успешно!"; } catch(Exception ex) { Label1.CssClass = "red"; Label1.Text = "Ошибка при отправке сообщения:<br />" + ex.Message; } } protected void Button2_Click(object sender, EventArgs e) { DropDownList1.ClearSelection(); TextBox1.Text = string.Empty; TextBox2.Text = string.Empty; TextBox3.Text = string.Empty; Label1.Text = string.Empty; } }
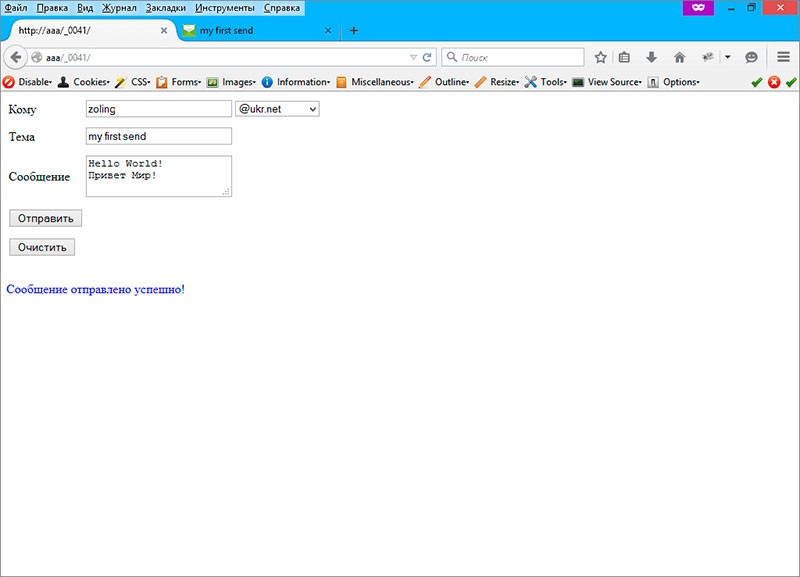