привязка одного значения
привязка нескольких значений
привязка объектов
привязка значений из базы данных
сложная привязка
привязка нескольких значений
привязка объектов
привязка значений из базы данных
сложная привязка
привязка одного значения
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Label ID="Label1" runat="server" Text="<%# str %>"></asp:Label> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Label ID="Label1" runat="server" Text="<%# str %>"></asp:Label> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected string str = "Hello World!"; protected void Page_Load(object sender, EventArgs e) { if(!IsPostBack) { Label1.DataBind(); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected string str = "Hello World!"; protected void Page_Load(object sender, EventArgs e) { if(!IsPostBack) { Label1.DataBind(); } } }
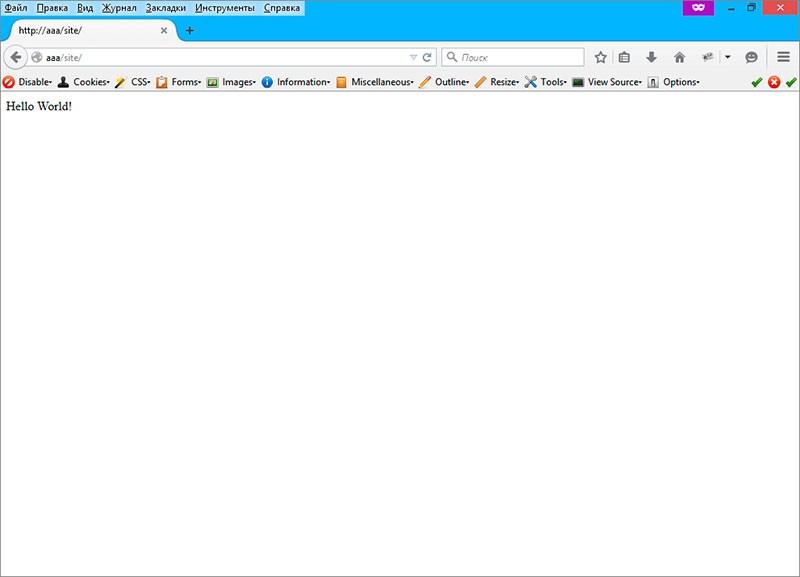
привязка нескольких значений
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server"></asp:ListBox> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server"></asp:ListBox> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { List<string> colors = new List<string>() { "Red", "Green", "Blue" }; protected void Page_Load(object sender, EventArgs e) { if(!IsPostBack) { ListBox1.DataSource = colors; ListBox1.DataBind(); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { List<string> colors = new List<string>() { "Red", "Green", "Blue" }; protected void Page_Load(object sender, EventArgs e) { if(!IsPostBack) { ListBox1.DataSource = colors; ListBox1.DataBind(); } } }
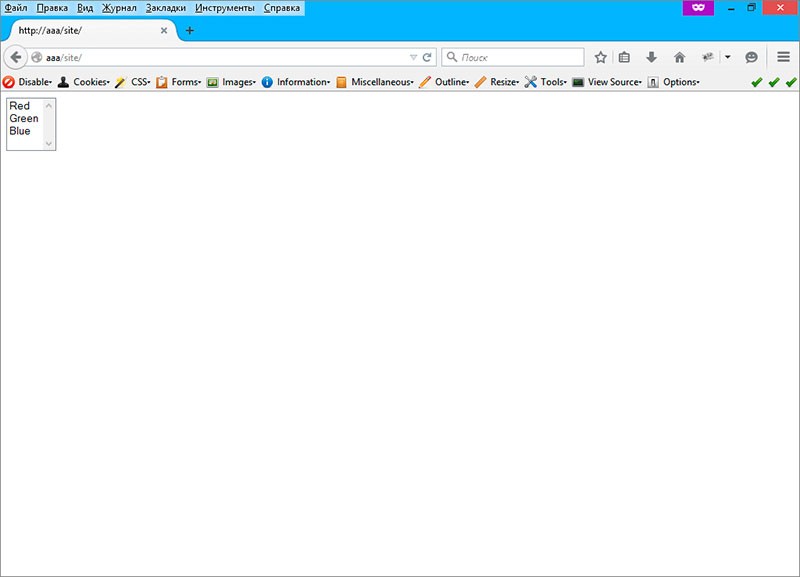
привязка объектов
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server"></asp:ListBox> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server"></asp:ListBox> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class myColor { string name; string hex; string rgb; public myColor(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } public partial class _Default : System.Web.UI.Page { List<myColor> colors = new List<myColor>(); protected void Page_Load(object sender, EventArgs e) { if(IsPostBack) { return; } colors.Add(new myColor("Red", "#FF0000", "255, 0, 0")); colors.Add(new myColor("Green", "#00FF00", "0, 255, 0")); colors.Add(new myColor("Blue", "#0000FF", "0, 0, 255")); ListBox1.DataSource = colors; /*текст у элемента управления*/ ListBox1.DataTextField = "Name"; /*значение у элемента управления*/ ListBox1.DataValueField = "Hex"; ListBox1.DataBind(); } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; ListBox1.ClearSelection(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class myColor { string name; string hex; string rgb; public myColor(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } public partial class _Default : System.Web.UI.Page { List<myColor> colors = new List<myColor>(); protected void Page_Load(object sender, EventArgs e) { if(IsPostBack) { return; } colors.Add(new myColor("Red", "#FF0000", "255, 0, 0")); colors.Add(new myColor("Green", "#00FF00", "0, 255, 0")); colors.Add(new myColor("Blue", "#0000FF", "0, 0, 255")); ListBox1.DataSource = colors; /*текст у элемента управления*/ ListBox1.DataTextField = "Name"; /*значение у элемента управления*/ ListBox1.DataValueField = "Hex"; ListBox1.DataBind(); } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; ListBox1.ClearSelection(); } }
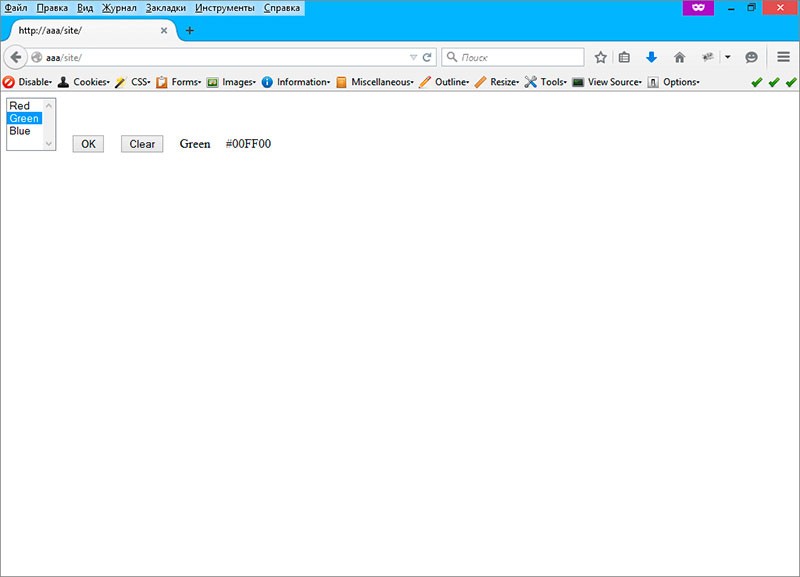
привязка значений из базы данных
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server" DataSourceID="SqlDataSource1" DataTextField="Name" DataValueField="Hex"></asp:ListBox> <asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [Name], [Hex] FROM [Colors]"></asp:SqlDataSource> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:ListBox ID="ListBox1" runat="server" DataSourceID="SqlDataSource1" DataTextField="Name" DataValueField="Hex"></asp:ListBox> <asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [Name], [Hex] FROM [Colors]"></asp:SqlDataSource> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; ListBox1.ClearSelection(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; ListBox1.ClearSelection(); } }
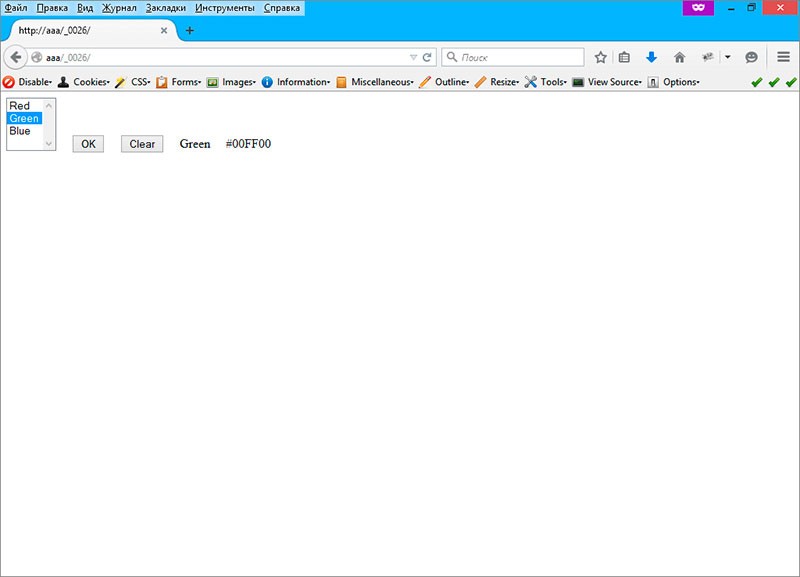
сложная привязка
Элемент управления ListBox будем привязывать к таблице Months и элементу управления DropDownList. Выберите Choose Data Source.
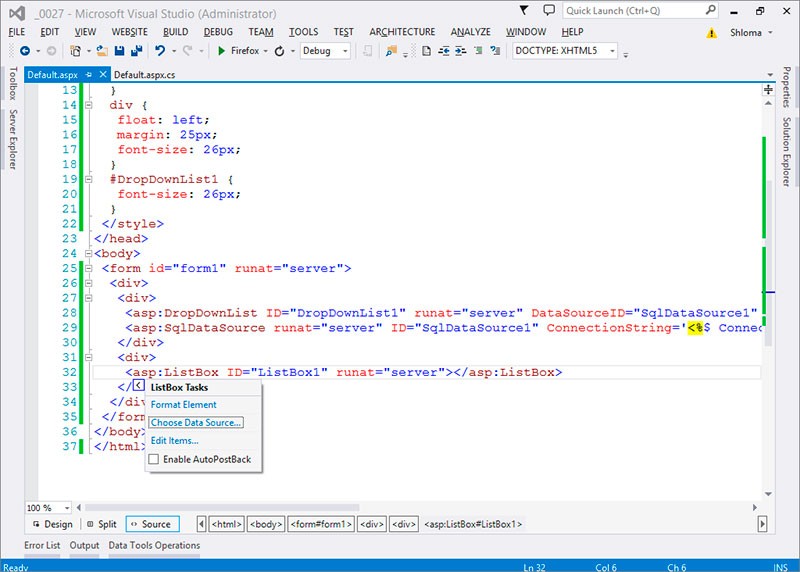
Выберите новый источник данных.
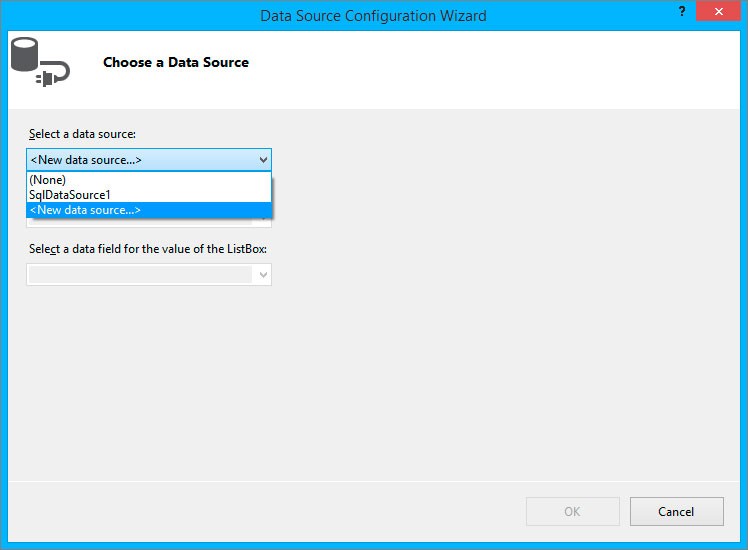
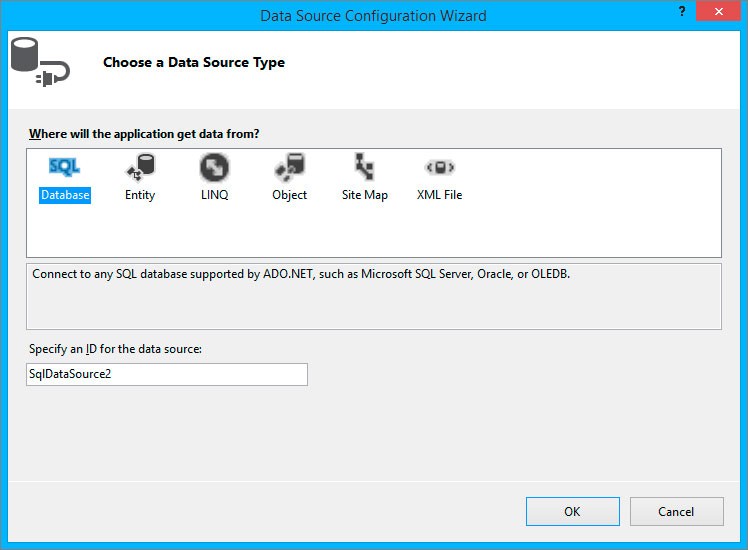
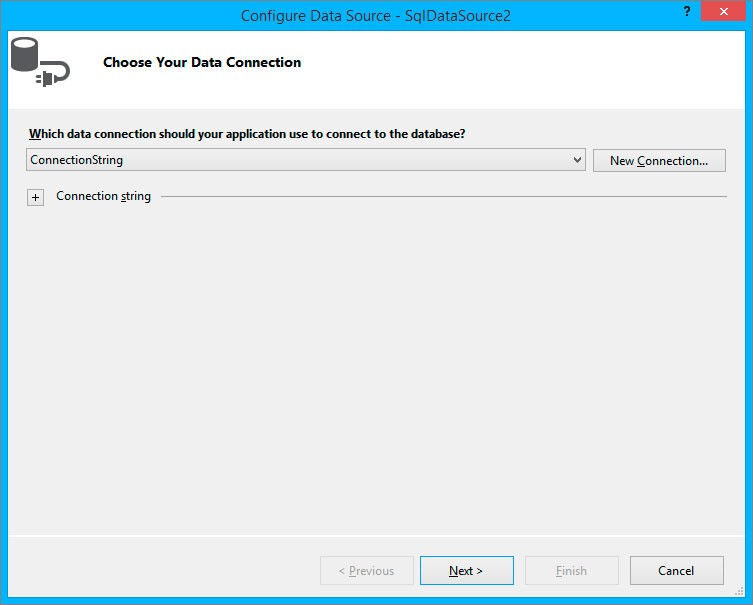
Выберите таблицу Months и два поля IDMonths и Month.
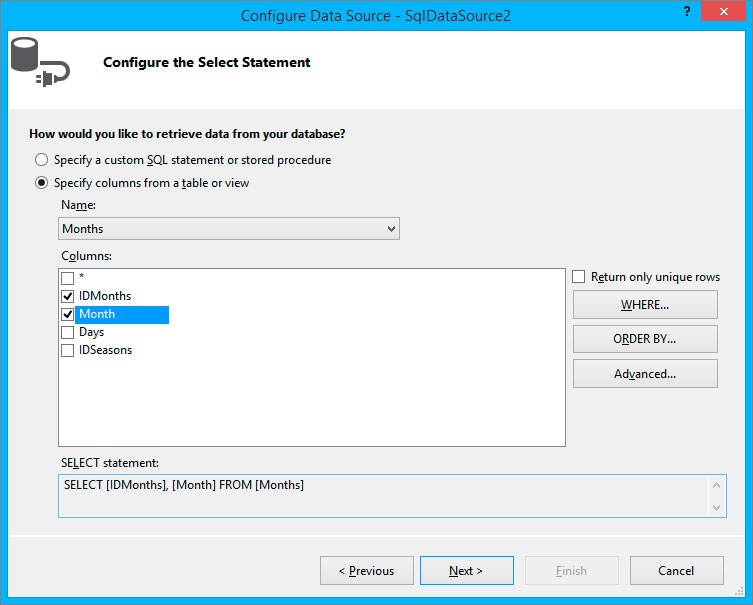
Теперь нужно создать условие, при котором выбранные поля будут иметь IDSeasons, выбранный в элементе управления DropDownList, к которому привязана таблица Seasons.
Нажмите на кнопку WHERE и из выпадающих списков выберите значения для запроса.
Нажмите на кнопку WHERE и из выпадающих списков выберите значения для запроса.
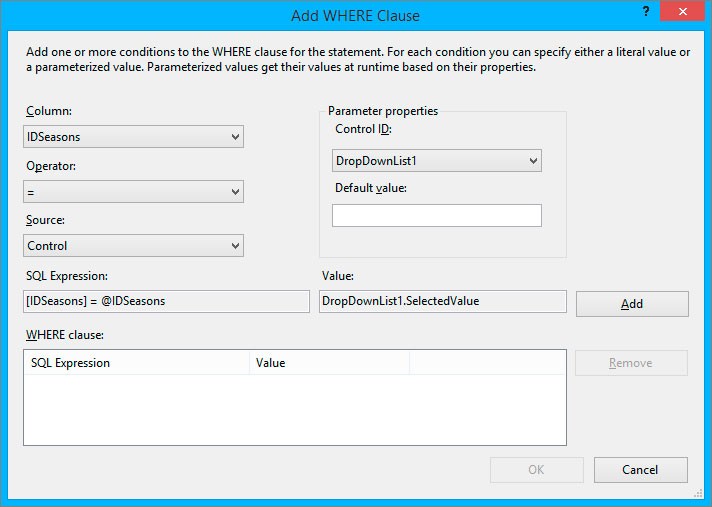
Нажмите на кнопку Add.
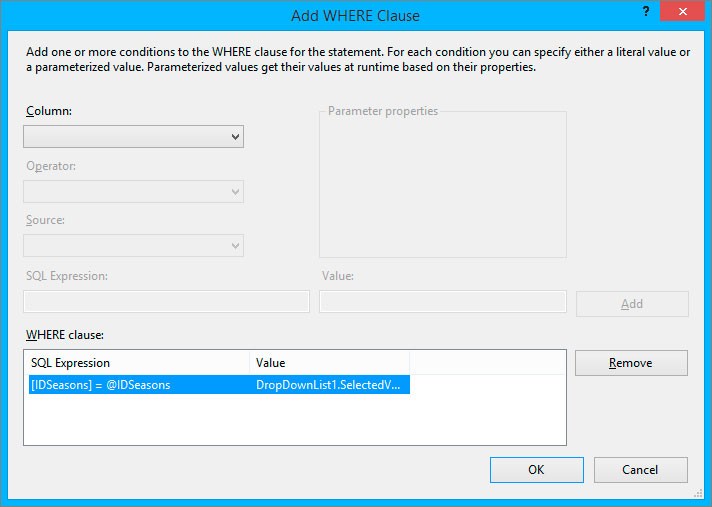
Произошло изменение запроса.
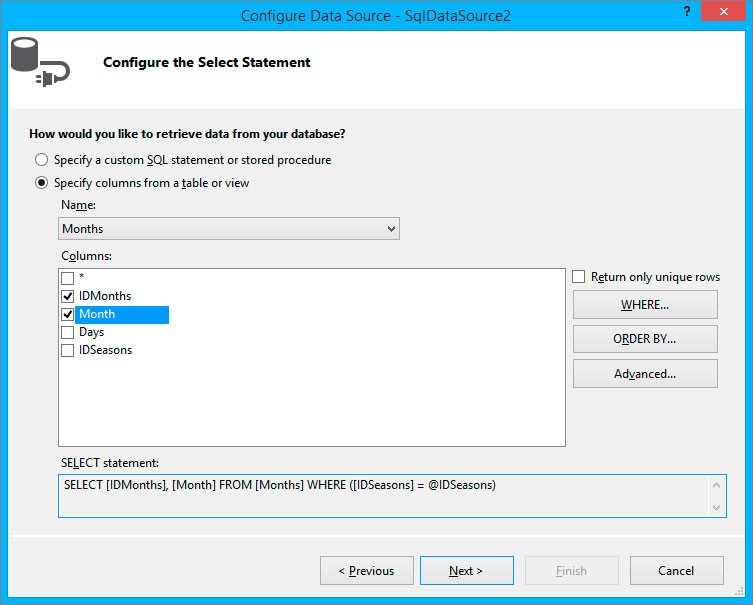
Нажмите Next.
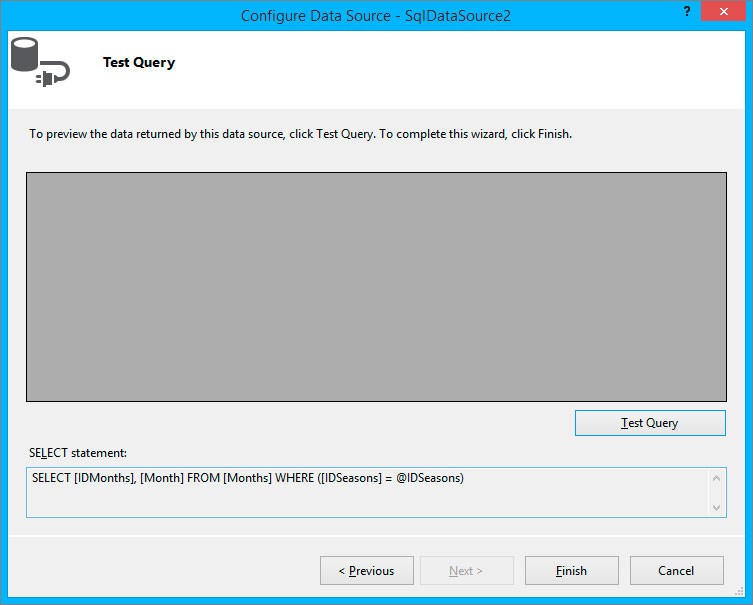
Нажмите на кнопку Test Query.
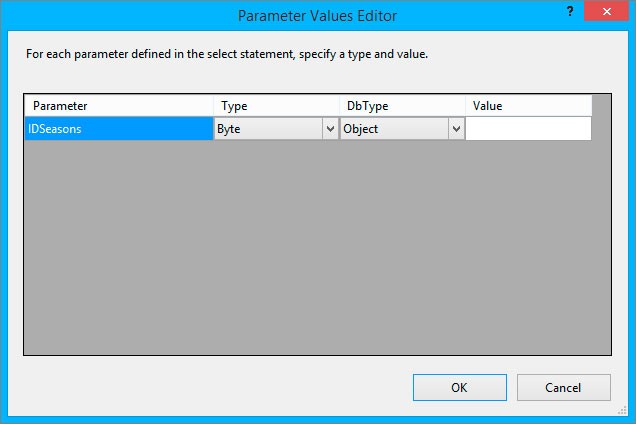
В поле Value введите значение, соответствующее IDSeasons, например 1 и нажмите OK.
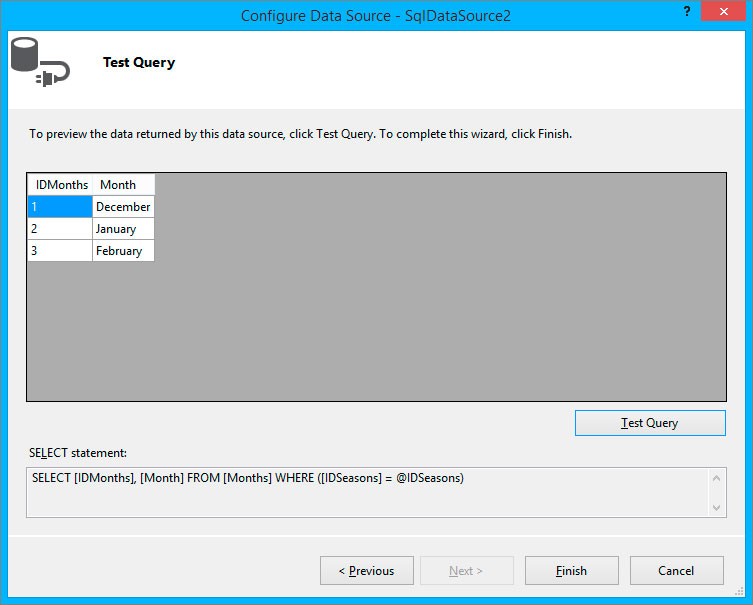
Нажмите на кнопку Finish. Выберите для текста столбец Month.
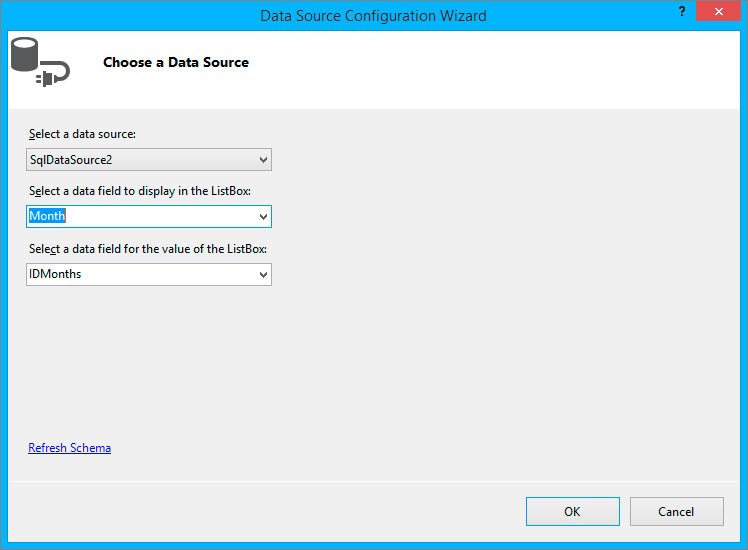
Для элемента управления DropDownList установите AutoPostBack в значение true или поставьте галочку.
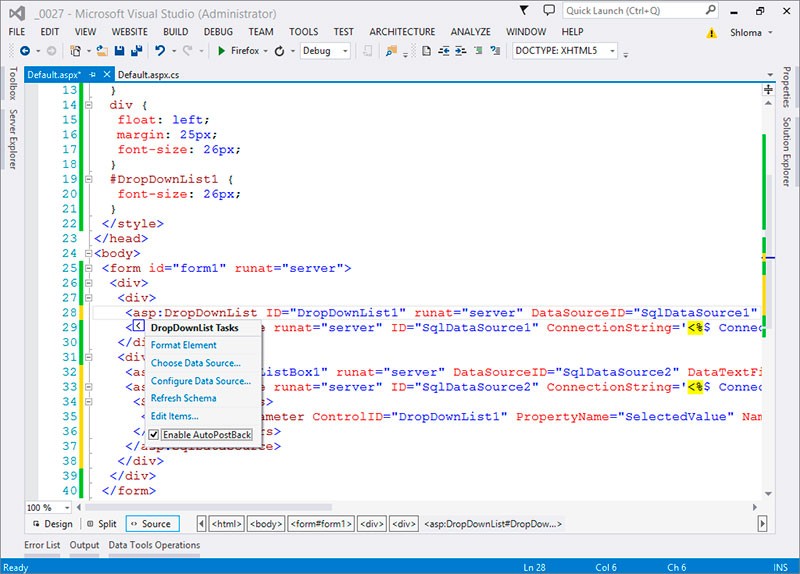
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0027.Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> div { float: left; margin: 10px; } #DropDownList1, #Button1, #Button2, #Label1, #Label2 { font-size: 24px; } #ListBox1 { font-size: 24px; width: 175px; } </style> </head> <body> <form id="form1" runat="server"> <div> <div> <asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="True" DataSourceID="SqlDataSource1" DataTextField="Season" DataValueField="IDSeasons"></asp:DropDownList> <asp:SqlDataSource runat="server" ID="SqlDataSource1" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT [Season], [IDSeasons] FROM [Seasons]"></asp:SqlDataSource> </div> <div> <asp:ListBox ID="ListBox1" runat="server" DataSourceID="SqlDataSource2" DataTextField="Month" DataValueField="Days"></asp:ListBox> <asp:SqlDataSource runat="server" ID="SqlDataSource2" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT [Month], [Days] FROM [Months] WHERE ([IDSeasons] = @IDSeasons)"> <SelectParameters> <asp:ControlParameter ControlID="DropDownList1" PropertyName="SelectedValue" Name="IDSeasons" Type="Int32"></asp:ControlParameter> </SelectParameters> </asp:SqlDataSource> </div> <div> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> </div> <div> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> </div> <div> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0027.Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> div { float: left; margin: 10px; } #DropDownList1, #Button1, #Button2, #Label1, #Label2 { font-size: 24px; } #ListBox1 { font-size: 24px; width: 175px; } </style> </head> <body> <form id="form1" runat="server"> <div> <div> <asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="True" DataSourceID="SqlDataSource1" DataTextField="Season" DataValueField="IDSeasons"></asp:DropDownList> <asp:SqlDataSource runat="server" ID="SqlDataSource1" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT [Season], [IDSeasons] FROM [Seasons]"></asp:SqlDataSource> </div> <div> <asp:ListBox ID="ListBox1" runat="server" DataSourceID="SqlDataSource2" DataTextField="Month" DataValueField="Days"></asp:ListBox> <asp:SqlDataSource runat="server" ID="SqlDataSource2" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT [Month], [Days] FROM [Months] WHERE ([IDSeasons] = @IDSeasons)"> <SelectParameters> <asp:ControlParameter ControlID="DropDownList1" PropertyName="SelectedValue" Name="IDSeasons" Type="Int32"></asp:ControlParameter> </SelectParameters> </asp:SqlDataSource> </div> <div> <asp:Button ID="Button1" runat="server" Text="OK" OnClick="Button1_Click" /> </div> <div> <asp:Button ID="Button2" runat="server" Text="Clear" OnClick="Button2_Click" /> </div> <div> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> <asp:Label ID="Label2" runat="server" Text=""></asp:Label> </div> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace _0027 { public partial class Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace _0027 { public partial class Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void Button1_Click(object sender, EventArgs e) { Label1.Text = ListBox1.SelectedItem.Text; Label2.Text = ListBox1.SelectedItem.Value; } protected void Button2_Click(object sender, EventArgs e) { Label1.Text = string.Empty; Label2.Text = string.Empty; } } }
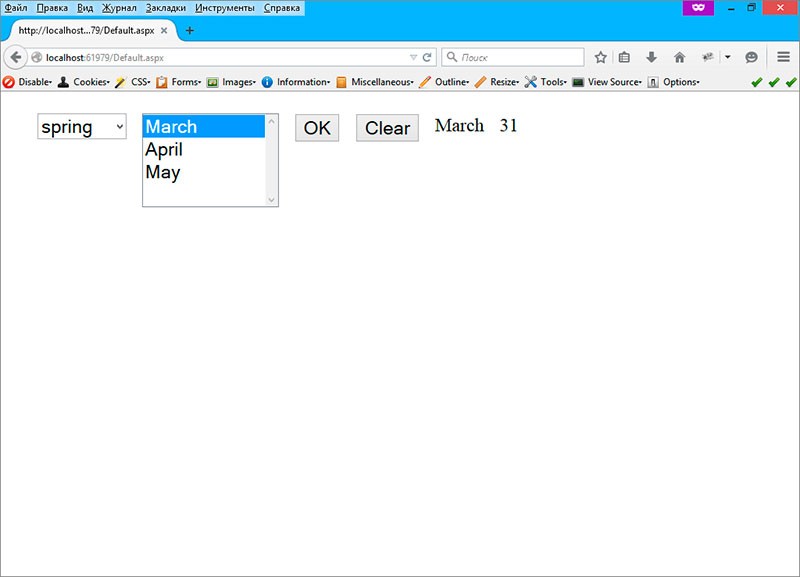