привязка к коллекции объектов
настраиваем колонки самостоятельно
привязка к базе данных
событие RowDataBound
извлечение данных из строки
настраиваем колонки самостоятельно
привязка к базе данных
событие RowDataBound
извлечение данных из строки
привязка к коллекции объектов
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:GridView ID="GridView1" runat="server"></asp:GridView> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:GridView ID="GridView1" runat="server"></asp:GridView> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); GridView1.DataSource = Colors; GridView1.DataBind(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); GridView1.DataSource = Colors; GridView1.DataBind(); } }
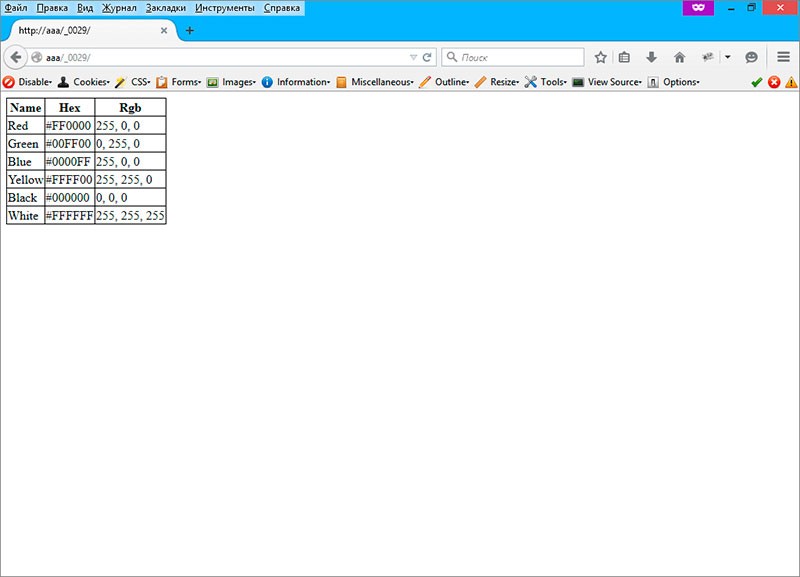
настраиваем колонки самостоятельно
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <%-- отключить AutoGenerateColumns="false" --%> <asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"> <Columns> <%-- DataField имя свойства из источника данных --%> <%-- HeaderText отображает название колонки --%> <asp:BoundField DataField="Name" HeaderText="Социальная сеть" /> <%-- DataImageUrlField имя свойства из источника данных --%> <%-- HeaderText отображает название колонки --%> <asp:ImageField DataImageUrlField="Image" HeaderText="Логотип"></asp:ImageField> <%-- DataNavigateUrlFields имя свойства из источника данных --%> <%-- DataTextField отображает текст <a>текст</a> --%> <%-- HeaderText отображает название колонки --%> <asp:HyperLinkField DataNavigateUrlFields="Url" DataTextField="Url" HeaderText="Ссылка" Target="_blank" /> </Columns> </asp:GridView> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <%-- отключить AutoGenerateColumns="false" --%> <asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"> <Columns> <%-- DataField имя свойства из источника данных --%> <%-- HeaderText отображает название колонки --%> <asp:BoundField DataField="Name" HeaderText="Социальная сеть" /> <%-- DataImageUrlField имя свойства из источника данных --%> <%-- HeaderText отображает название колонки --%> <asp:ImageField DataImageUrlField="Image" HeaderText="Логотип"></asp:ImageField> <%-- DataNavigateUrlFields имя свойства из источника данных --%> <%-- DataTextField отображает текст <a>текст</a> --%> <%-- HeaderText отображает название колонки --%> <asp:HyperLinkField DataNavigateUrlFields="Url" DataTextField="Url" HeaderText="Ссылка" Target="_blank" /> </Columns> </asp:GridView> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class SocialNetworks { string name; string image; string url; public SocialNetworks(string arg0, string arg1, string arg2) { name = arg0; image = arg1; url = arg2; } public string Name { get { return name; } } public string Image { get { return image; } } public string Url { get { return url; } } } public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<SocialNetworks> Networks = new List<SocialNetworks>(); Networks.Add(new SocialNetworks("Facebook", "img/facebook.png", "https://www.facebook.com/")); Networks.Add(new SocialNetworks("Googleplus", "img/googleplus.png", "https://plus.google.com/")); Networks.Add(new SocialNetworks("Twitter", "img/twitter.png", "https://twitter.com/")); Networks.Add(new SocialNetworks("Vkontakte", "img/vkontakte.png", "https://vk.com/")); Networks.Add(new SocialNetworks("Yahoo", "img/yahoo.png", "https://www.yahoo.com/")); GridView1.DataSource = Networks; GridView1.DataBind(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; class SocialNetworks { string name; string image; string url; public SocialNetworks(string arg0, string arg1, string arg2) { name = arg0; image = arg1; url = arg2; } public string Name { get { return name; } } public string Image { get { return image; } } public string Url { get { return url; } } } public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<SocialNetworks> Networks = new List<SocialNetworks>(); Networks.Add(new SocialNetworks("Facebook", "img/facebook.png", "https://www.facebook.com/")); Networks.Add(new SocialNetworks("Googleplus", "img/googleplus.png", "https://plus.google.com/")); Networks.Add(new SocialNetworks("Twitter", "img/twitter.png", "https://twitter.com/")); Networks.Add(new SocialNetworks("Vkontakte", "img/vkontakte.png", "https://vk.com/")); Networks.Add(new SocialNetworks("Yahoo", "img/yahoo.png", "https://www.yahoo.com/")); GridView1.DataSource = Networks; GridView1.DataBind(); } }
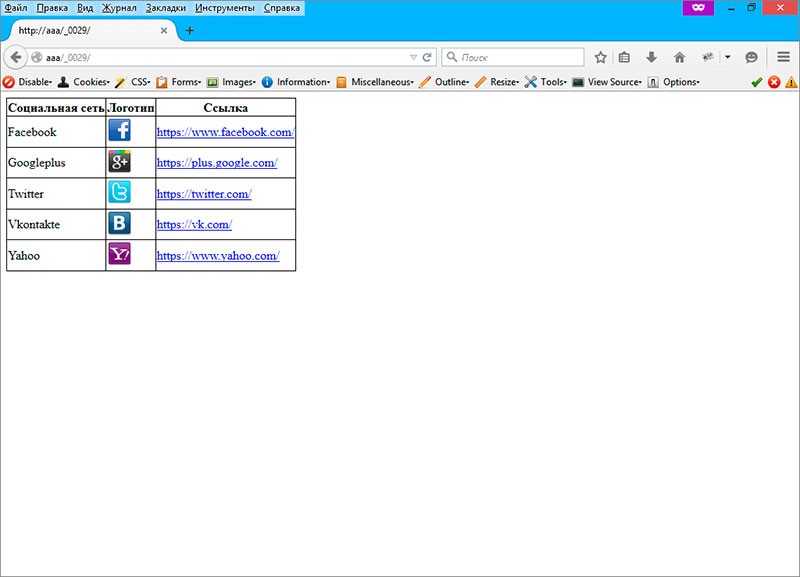
привязка к базе данных
Для операции редактирования и удаления, нажмите кнопку Advanced.
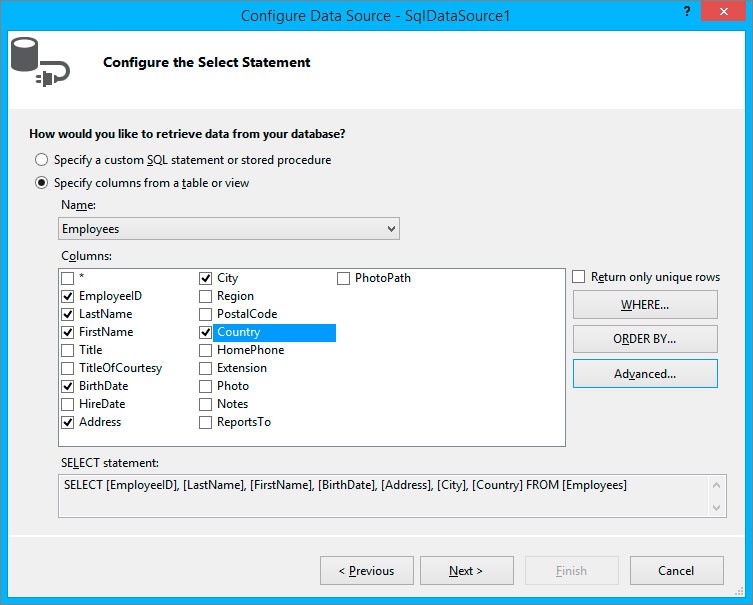
Выберите опцию Generate INSETR, UPDATE and DELETE statements.
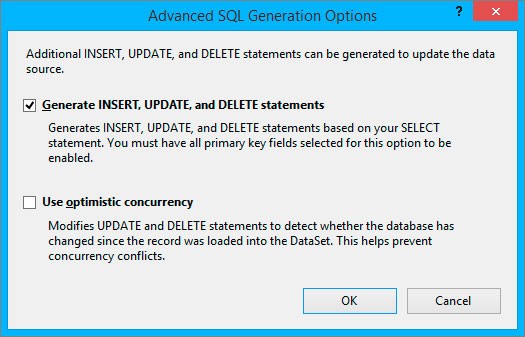
Подключите эти опции.
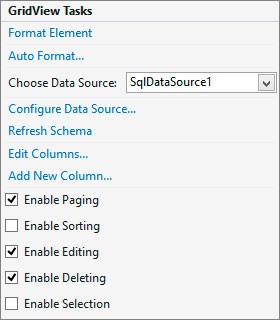
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0030.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
-
<
meta
charset
=
"utf-8"
/>
-
<
title
></
title
>
</
head
>
<
body
>
-
<
form
id
=
"form1"
runat
=
"server"
>
-
<
div
>
-
<%-- PageSize="5" будет отображать 5 строк на 1 странице --%>
-
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AutoGenerateColumns
=
"False"
DataKeyNames
=
"EmployeeID"
DataSourceID
=
"SqlDataSource1"
AllowPaging
=
"True"
PageSize
=
"5"
>
-
<
Columns
>
-
<
asp:CommandField
ShowEditButton
=
"True"
ShowDeleteButton
=
"True"
></
asp:CommandField
>
-
<
asp:BoundField
DataField
=
"EmployeeID"
HeaderText
=
"EmployeeID"
ReadOnly
=
"True"
InsertVisible
=
"False"
SortExpression
=
"EmployeeID"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"LastName"
HeaderText
=
"LastName"
SortExpression
=
"LastName"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"FirstName"
HeaderText
=
"FirstName"
SortExpression
=
"FirstName"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"BirthDate"
HeaderText
=
"BirthDate"
SortExpression
=
"BirthDate"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"Address"
HeaderText
=
"Address"
SortExpression
=
"Address"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"City"
HeaderText
=
"City"
SortExpression
=
"City"
></
asp:BoundField
>
-
<
asp:BoundField
DataField
=
"Country"
HeaderText
=
"Country"
SortExpression
=
"Country"
></
asp:BoundField
>
-
</
Columns
>
-
</
asp:GridView
>
-
<
asp:SqlDataSource
runat
=
"server"
ID
=
"SqlDataSource1"
ConnectionString='<%$ ConnectionStrings:ConnectionString %>' DeleteCommand="DELETE FROM [Employees] WHERE [EmployeeID] = @EmployeeID" InsertCommand="INSERT INTO [Employees] ([LastName], [FirstName], [BirthDate], [Address], [City], [Country]) VALUES (@LastName, @FirstName, @BirthDate, @Address, @City, @Country)" SelectCommand="SELECT [EmployeeID], [LastName], [FirstName], [BirthDate], [Address], [City], [Country] FROM [Employees]" UpdateCommand="UPDATE [Employees] SET [LastName] = @LastName, [FirstName] = @FirstName, [BirthDate] = @BirthDate, [Address] = @Address, [City] = @City, [Country] = @Country WHERE [EmployeeID] = @EmployeeID">
-
<
DeleteParameters
>
-
<
asp:Parameter
Name
=
"EmployeeID"
Type
=
"Int32"
></
asp:Parameter
>
-
</
DeleteParameters
>
-
<
InsertParameters
>
-
<
asp:Parameter
Name
=
"LastName"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"FirstName"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"BirthDate"
Type
=
"DateTime"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"Address"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"City"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"Country"
Type
=
"String"
></
asp:Parameter
>
-
</
InsertParameters
>
-
<
UpdateParameters
>
-
<
asp:Parameter
Name
=
"LastName"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"FirstName"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"BirthDate"
Type
=
"DateTime"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"Address"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"City"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"Country"
Type
=
"String"
></
asp:Parameter
>
-
<
asp:Parameter
Name
=
"EmployeeID"
Type
=
"Int32"
></
asp:Parameter
>
-
</
UpdateParameters
>
-
</
asp:SqlDataSource
>
-
</
div
>
-
</
form
>
</
body
>
</
html
>
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0030.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
<
meta
charset
=
"utf-8"
/>
<
title
></
title
>
</
head
>
<
body
>
<
form
id
=
"form1"
runat
=
"server"
>
<
div
>
<%-- PageSize="5" будет отображать 5 строк на 1 странице --%>
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AutoGenerateColumns
=
"False"
DataKeyNames
=
"EmployeeID"
DataSourceID
=
"SqlDataSource1"
AllowPaging
=
"True"
PageSize
=
"5"
>
<
Columns
>
<
asp:CommandField
ShowEditButton
=
"True"
ShowDeleteButton
=
"True"
></
asp:CommandField
>
<
asp:BoundField
DataField
=
"EmployeeID"
HeaderText
=
"EmployeeID"
ReadOnly
=
"True"
InsertVisible
=
"False"
SortExpression
=
"EmployeeID"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"LastName"
HeaderText
=
"LastName"
SortExpression
=
"LastName"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"FirstName"
HeaderText
=
"FirstName"
SortExpression
=
"FirstName"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"BirthDate"
HeaderText
=
"BirthDate"
SortExpression
=
"BirthDate"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"Address"
HeaderText
=
"Address"
SortExpression
=
"Address"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"City"
HeaderText
=
"City"
SortExpression
=
"City"
></
asp:BoundField
>
<
asp:BoundField
DataField
=
"Country"
HeaderText
=
"Country"
SortExpression
=
"Country"
></
asp:BoundField
>
</
Columns
>
</
asp:GridView
>
<
asp:SqlDataSource
runat
=
"server"
ID
=
"SqlDataSource1"
ConnectionString='<%$ ConnectionStrings:ConnectionString %>' DeleteCommand="DELETE FROM [Employees] WHERE [EmployeeID] = @EmployeeID" InsertCommand="INSERT INTO [Employees] ([LastName], [FirstName], [BirthDate], [Address], [City], [Country]) VALUES (@LastName, @FirstName, @BirthDate, @Address, @City, @Country)" SelectCommand="SELECT [EmployeeID], [LastName], [FirstName], [BirthDate], [Address], [City], [Country] FROM [Employees]" UpdateCommand="UPDATE [Employees] SET [LastName] = @LastName, [FirstName] = @FirstName, [BirthDate] = @BirthDate, [Address] = @Address, [City] = @City, [Country] = @Country WHERE [EmployeeID] = @EmployeeID">
<
DeleteParameters
>
<
asp:Parameter
Name
=
"EmployeeID"
Type
=
"Int32"
></
asp:Parameter
>
</
DeleteParameters
>
<
InsertParameters
>
<
asp:Parameter
Name
=
"LastName"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"FirstName"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"BirthDate"
Type
=
"DateTime"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"Address"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"City"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"Country"
Type
=
"String"
></
asp:Parameter
>
</
InsertParameters
>
<
UpdateParameters
>
<
asp:Parameter
Name
=
"LastName"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"FirstName"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"BirthDate"
Type
=
"DateTime"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"Address"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"City"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"Country"
Type
=
"String"
></
asp:Parameter
>
<
asp:Parameter
Name
=
"EmployeeID"
Type
=
"Int32"
></
asp:Parameter
>
</
UpdateParameters
>
</
asp:SqlDataSource
>
</
div
>
</
form
>
</
body
>
</
html
>
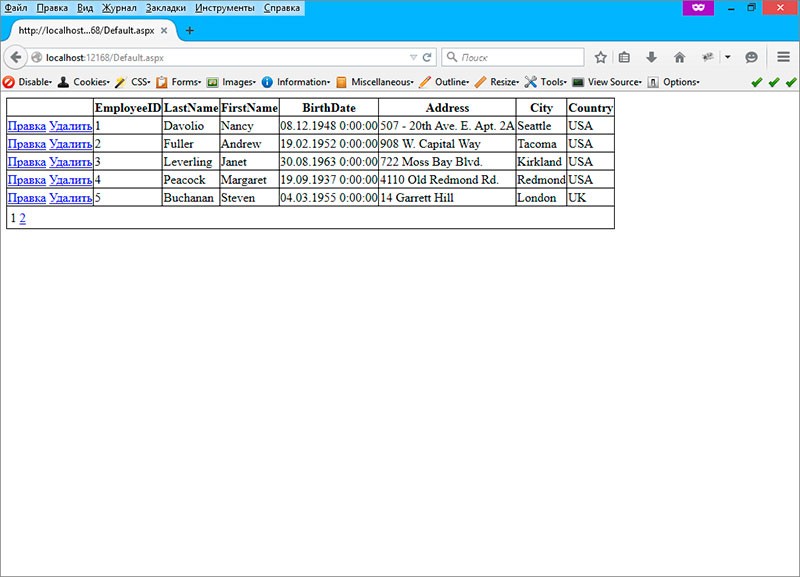
событие RowDataBound
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0031.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
-
<
meta
charset
=
"utf-8"
/>
-
<
title
></
title
>
-
<
style
>
-
.usa {
-
background-color: #ff9ad1;
-
}
-
.germany {
-
background-color: #a8e992;
-
}
-
.france {
-
background-color: #a8d3ff;
-
}
-
</
style
>
</
head
>
<
body
>
-
<
form
id
=
"form1"
runat
=
"server"
>
-
<
div
>
-
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AllowPaging
=
"True"
AutoGenerateColumns
=
"False"
DataSourceID
=
"SqlDataSource1"
PageSize
=
"10"
OnRowDataBound
=
"GridView1_RowDataBound"
>
-
<
Columns
>
-
<
asp:BoundField
DataField
=
"CompanyName"
HeaderText
=
"CompanyName"
SortExpression
=
"CompanyName"
/>
-
<
asp:BoundField
DataField
=
"Address"
HeaderText
=
"Address"
SortExpression
=
"Address"
/>
-
<
asp:BoundField
DataField
=
"City"
HeaderText
=
"City"
SortExpression
=
"City"
/>
-
<
asp:BoundField
DataField
=
"Country"
HeaderText
=
"Country"
SortExpression
=
"Country"
/>
-
<
asp:BoundField
DataField
=
"Phone"
HeaderText
=
"Phone"
SortExpression
=
"Phone"
/>
-
</
Columns
>
-
</
asp:GridView
>
-
<
asp:SqlDataSource
ID
=
"SqlDataSource1"
runat
=
"server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [CompanyName], [Address], [City], [Country], [Phone] FROM [Customers]"></
asp:SqlDataSource
>
-
</
div
>
-
</
form
>
</
body
>
</
html
>
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0031.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
<
meta
charset
=
"utf-8"
/>
<
title
></
title
>
<
style
>
.usa {
background-color: #ff9ad1;
}
.germany {
background-color: #a8e992;
}
.france {
background-color: #a8d3ff;
}
</
style
>
</
head
>
<
body
>
<
form
id
=
"form1"
runat
=
"server"
>
<
div
>
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AllowPaging
=
"True"
AutoGenerateColumns
=
"False"
DataSourceID
=
"SqlDataSource1"
PageSize
=
"10"
OnRowDataBound
=
"GridView1_RowDataBound"
>
<
Columns
>
<
asp:BoundField
DataField
=
"CompanyName"
HeaderText
=
"CompanyName"
SortExpression
=
"CompanyName"
/>
<
asp:BoundField
DataField
=
"Address"
HeaderText
=
"Address"
SortExpression
=
"Address"
/>
<
asp:BoundField
DataField
=
"City"
HeaderText
=
"City"
SortExpression
=
"City"
/>
<
asp:BoundField
DataField
=
"Country"
HeaderText
=
"Country"
SortExpression
=
"Country"
/>
<
asp:BoundField
DataField
=
"Phone"
HeaderText
=
"Phone"
SortExpression
=
"Phone"
/>
</
Columns
>
</
asp:GridView
>
<
asp:SqlDataSource
ID
=
"SqlDataSource1"
runat
=
"server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [CompanyName], [Address], [City], [Country], [Phone] FROM [Customers]"></
asp:SqlDataSource
>
</
div
>
</
form
>
</
body
>
</
html
>
Default.aspx.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
namespace
_0031 {
-
public
partial
class
Default : System.Web.UI.Page {
-
protected
void
Page_Load(
object
sender, EventArgs e) {
-
}
-
protected
void
GridView1_RowDataBound(
object
sender, GridViewRowEventArgs e) {
-
/*проверяем, какая часть GridView1 формируется*/
-
/*DataRow - это строка с данными*/
-
if
(e.Row.RowType == DataControlRowType.DataRow) {
-
/*DataBinder.Eval() - получаем значение свойства из указанного объекта*/
-
string
str = (
string
)DataBinder.Eval(e.Row.DataItem,
"Country"
);
-
if
(str ==
"USA"
) {
-
e.Row.CssClass =
"usa"
;
-
}
-
else
if
(str ==
"Germany"
) {
-
e.Row.CssClass =
"germany"
;
-
}
-
else
if
(str ==
"France"
) {
-
e.Row.CssClass =
"france"
;
-
}
-
}
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
namespace
_0031 {
public
partial
class
Default : System.Web.UI.Page {
protected
void
Page_Load(
object
sender, EventArgs e) {
}
protected
void
GridView1_RowDataBound(
object
sender, GridViewRowEventArgs e) {
/*проверяем, какая часть GridView1 формируется*/
/*DataRow - это строка с данными*/
if
(e.Row.RowType == DataControlRowType.DataRow) {
/*DataBinder.Eval() - получаем значение свойства из указанного объекта*/
string
str = (
string
)DataBinder.Eval(e.Row.DataItem,
"Country"
);
if
(str ==
"USA"
) {
e.Row.CssClass =
"usa"
;
}
else
if
(str ==
"Germany"
) {
e.Row.CssClass =
"germany"
;
}
else
if
(str ==
"France"
) {
e.Row.CssClass =
"france"
;
}
}
}
}
}
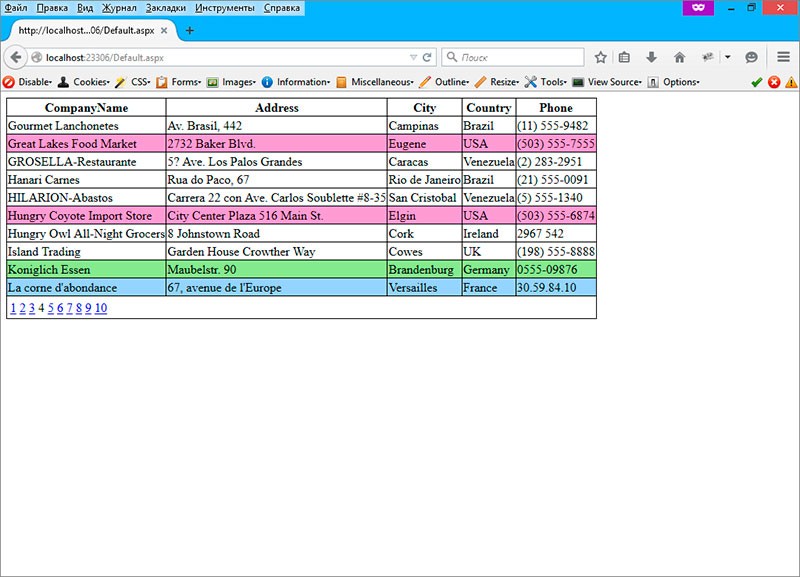
извлечение данных из строки
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0032.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
-
<
meta
charset
=
"utf-8"
/>
-
<
title
></
title
>
</
head
>
<
body
>
-
<
form
id
=
"form1"
runat
=
"server"
>
-
<
div
>
-
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AllowPaging
=
"True"
AutoGenerateColumns
=
"False"
DataKeyNames
=
"ProductID"
DataSourceID
=
"SqlDataSource1"
CellPadding
=
"4"
ForeColor
=
"#333333"
GridLines
=
"None"
OnSelectedIndexChanged
=
"GridView1_SelectedIndexChanged"
>
-
<
AlternatingRowStyle
BackColor
=
"White"
/>
-
<
Columns
>
-
<
asp:CommandField
ShowDeleteButton
=
"True"
ShowEditButton
=
"True"
ShowSelectButton
=
"True"
/>
-
<
asp:BoundField
DataField
=
"ProductID"
HeaderText
=
"ProductID"
ReadOnly
=
"True"
SortExpression
=
"ProductID"
InsertVisible
=
"False"
/>
-
<
asp:BoundField
DataField
=
"ProductName"
HeaderText
=
"ProductName"
SortExpression
=
"ProductName"
/>
-
<
asp:BoundField
DataField
=
"QuantityPerUnit"
HeaderText
=
"QuantityPerUnit"
SortExpression
=
"QuantityPerUnit"
/>
-
<
asp:BoundField
DataField
=
"UnitPrice"
HeaderText
=
"UnitPrice"
SortExpression
=
"UnitPrice"
/>
-
</
Columns
>
-
<
EditRowStyle
BackColor
=
"#2461BF"
/>
-
<
FooterStyle
BackColor
=
"#507CD1"
Font-Bold
=
"True"
ForeColor
=
"White"
/>
-
<
HeaderStyle
BackColor
=
"#507CD1"
Font-Bold
=
"True"
ForeColor
=
"White"
/>
-
<
PagerStyle
BackColor
=
"#2461BF"
ForeColor
=
"White"
HorizontalAlign
=
"Center"
/>
-
<
RowStyle
BackColor
=
"#EFF3FB"
/>
-
<
SelectedRowStyle
BackColor
=
"#D1DDF1"
Font-Bold
=
"True"
ForeColor
=
"#333333"
/>
-
<
SortedAscendingCellStyle
BackColor
=
"#F5F7FB"
/>
-
<
SortedAscendingHeaderStyle
BackColor
=
"#6D95E1"
/>
-
<
SortedDescendingCellStyle
BackColor
=
"#E9EBEF"
/>
-
<
SortedDescendingHeaderStyle
BackColor
=
"#4870BE"
/>
-
</
asp:GridView
>
-
<
asp:SqlDataSource
ID
=
"SqlDataSource1"
runat
=
"server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [ProductID], [ProductName], [QuantityPerUnit], [UnitPrice] FROM [Products]" DeleteCommand="DELETE FROM [Products] WHERE [ProductID] = @ProductID" InsertCommand="INSERT INTO [Products] ([ProductName], [QuantityPerUnit], [UnitPrice]) VALUES (@ProductName, @QuantityPerUnit, @UnitPrice)" UpdateCommand="UPDATE [Products] SET [ProductName] = @ProductName, [QuantityPerUnit] = @QuantityPerUnit, [UnitPrice] = @UnitPrice WHERE [ProductID] = @ProductID">
-
<
DeleteParameters
>
-
<
asp:Parameter
Name
=
"ProductID"
Type
=
"Int32"
/>
-
</
DeleteParameters
>
-
<
InsertParameters
>
-
<
asp:Parameter
Name
=
"ProductName"
Type
=
"String"
/>
-
<
asp:Parameter
Name
=
"QuantityPerUnit"
Type
=
"String"
/>
-
<
asp:Parameter
Name
=
"UnitPrice"
Type
=
"Decimal"
/>
-
</
InsertParameters
>
-
<
UpdateParameters
>
-
<
asp:Parameter
Name
=
"ProductName"
Type
=
"String"
/>
-
<
asp:Parameter
Name
=
"QuantityPerUnit"
Type
=
"String"
/>
-
<
asp:Parameter
Name
=
"UnitPrice"
Type
=
"Decimal"
/>
-
<
asp:Parameter
Name
=
"ProductID"
Type
=
"Int32"
/>
-
</
UpdateParameters
>
-
</
asp:SqlDataSource
>
-
<
br
/>
-
<
br
/>
-
<
asp:Label
ID
=
"Label1"
runat
=
"server"
Text
=
""
></
asp:Label
>
-
</
div
>
-
</
form
>
</
body
>
</
html
>
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_0032.Default" %>
<!DOCTYPE html>
<
html
xmlns
=
"http://www.w3.org/1999/xhtml"
>
<
head
runat
=
"server"
>
<
meta
charset
=
"utf-8"
/>
<
title
></
title
>
</
head
>
<
body
>
<
form
id
=
"form1"
runat
=
"server"
>
<
div
>
<
asp:GridView
ID
=
"GridView1"
runat
=
"server"
AllowPaging
=
"True"
AutoGenerateColumns
=
"False"
DataKeyNames
=
"ProductID"
DataSourceID
=
"SqlDataSource1"
CellPadding
=
"4"
ForeColor
=
"#333333"
GridLines
=
"None"
OnSelectedIndexChanged
=
"GridView1_SelectedIndexChanged"
>
<
AlternatingRowStyle
BackColor
=
"White"
/>
<
Columns
>
<
asp:CommandField
ShowDeleteButton
=
"True"
ShowEditButton
=
"True"
ShowSelectButton
=
"True"
/>
<
asp:BoundField
DataField
=
"ProductID"
HeaderText
=
"ProductID"
ReadOnly
=
"True"
SortExpression
=
"ProductID"
InsertVisible
=
"False"
/>
<
asp:BoundField
DataField
=
"ProductName"
HeaderText
=
"ProductName"
SortExpression
=
"ProductName"
/>
<
asp:BoundField
DataField
=
"QuantityPerUnit"
HeaderText
=
"QuantityPerUnit"
SortExpression
=
"QuantityPerUnit"
/>
<
asp:BoundField
DataField
=
"UnitPrice"
HeaderText
=
"UnitPrice"
SortExpression
=
"UnitPrice"
/>
</
Columns
>
<
EditRowStyle
BackColor
=
"#2461BF"
/>
<
FooterStyle
BackColor
=
"#507CD1"
Font-Bold
=
"True"
ForeColor
=
"White"
/>
<
HeaderStyle
BackColor
=
"#507CD1"
Font-Bold
=
"True"
ForeColor
=
"White"
/>
<
PagerStyle
BackColor
=
"#2461BF"
ForeColor
=
"White"
HorizontalAlign
=
"Center"
/>
<
RowStyle
BackColor
=
"#EFF3FB"
/>
<
SelectedRowStyle
BackColor
=
"#D1DDF1"
Font-Bold
=
"True"
ForeColor
=
"#333333"
/>
<
SortedAscendingCellStyle
BackColor
=
"#F5F7FB"
/>
<
SortedAscendingHeaderStyle
BackColor
=
"#6D95E1"
/>
<
SortedDescendingCellStyle
BackColor
=
"#E9EBEF"
/>
<
SortedDescendingHeaderStyle
BackColor
=
"#4870BE"
/>
</
asp:GridView
>
<
asp:SqlDataSource
ID
=
"SqlDataSource1"
runat
=
"server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT [ProductID], [ProductName], [QuantityPerUnit], [UnitPrice] FROM [Products]" DeleteCommand="DELETE FROM [Products] WHERE [ProductID] = @ProductID" InsertCommand="INSERT INTO [Products] ([ProductName], [QuantityPerUnit], [UnitPrice]) VALUES (@ProductName, @QuantityPerUnit, @UnitPrice)" UpdateCommand="UPDATE [Products] SET [ProductName] = @ProductName, [QuantityPerUnit] = @QuantityPerUnit, [UnitPrice] = @UnitPrice WHERE [ProductID] = @ProductID">
<
DeleteParameters
>
<
asp:Parameter
Name
=
"ProductID"
Type
=
"Int32"
/>
</
DeleteParameters
>
<
InsertParameters
>
<
asp:Parameter
Name
=
"ProductName"
Type
=
"String"
/>
<
asp:Parameter
Name
=
"QuantityPerUnit"
Type
=
"String"
/>
<
asp:Parameter
Name
=
"UnitPrice"
Type
=
"Decimal"
/>
</
InsertParameters
>
<
UpdateParameters
>
<
asp:Parameter
Name
=
"ProductName"
Type
=
"String"
/>
<
asp:Parameter
Name
=
"QuantityPerUnit"
Type
=
"String"
/>
<
asp:Parameter
Name
=
"UnitPrice"
Type
=
"Decimal"
/>
<
asp:Parameter
Name
=
"ProductID"
Type
=
"Int32"
/>
</
UpdateParameters
>
</
asp:SqlDataSource
>
<
br
/>
<
br
/>
<
asp:Label
ID
=
"Label1"
runat
=
"server"
Text
=
""
></
asp:Label
>
</
div
>
</
form
>
</
body
>
</
html
>
Default.aspx.cs
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
namespace
_0032 {
-
public
partial
class
Default : System.Web.UI.Page {
-
protected
void
Page_Load(
object
sender, EventArgs e) {
-
}
-
protected
void
GridView1_SelectedIndexChanged(
object
sender, EventArgs e) {
-
/*номер строки на странице*/
-
int
numberLine = GridView1.SelectedIndex;
-
numberLine++;
-
int
ProductID = (
int
)GridView1.SelectedDataKey.Values[
"ProductID"
];
-
string
ProductName = GridView1.SelectedRow.Cells[1].Text;
-
string
QuantityPerUnit = GridView1.SelectedRow.Cells[2].Text;
-
string
UnitPrice = GridView1.SelectedRow.Cells[3].Text;
-
Label1.Text =
string
.Format(
"номер строки на странице : {0}<br />ID : {1}<br />название : {2}<br />количество : {3}<br />цена : {4}"
, numberLine, ProductID, ProductName, QuantityPerUnit, UnitPrice);
-
}
-
}
}
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
namespace
_0032 {
public
partial
class
Default : System.Web.UI.Page {
protected
void
Page_Load(
object
sender, EventArgs e) {
}
protected
void
GridView1_SelectedIndexChanged(
object
sender, EventArgs e) {
/*номер строки на странице*/
int
numberLine = GridView1.SelectedIndex;
numberLine++;
int
ProductID = (
int
)GridView1.SelectedDataKey.Values[
"ProductID"
];
string
ProductName = GridView1.SelectedRow.Cells[1].Text;
string
QuantityPerUnit = GridView1.SelectedRow.Cells[2].Text;
string
UnitPrice = GridView1.SelectedRow.Cells[3].Text;
Label1.Text =
string
.Format(
"номер строки на странице : {0}<br />ID : {1}<br />название : {2}<br />количество : {3}<br />цена : {4}"
, numberLine, ProductID, ProductName, QuantityPerUnit, UnitPrice);
}
}
}
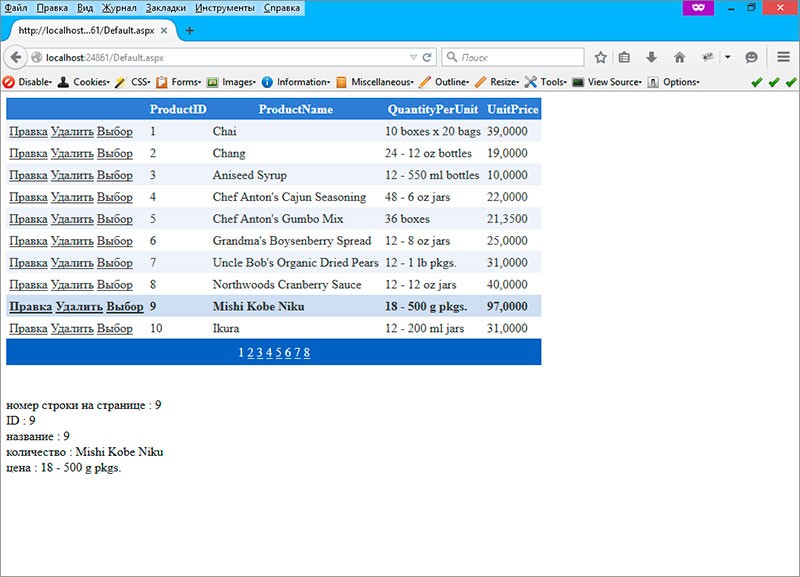