ViewState не использует ресурсов сервера, в браузер передается в виде скрытого поля input, а информация, закодированная с помощью Base64, записана в атрибуте value.
При закрытии страницы в браузере, информация не сохраняется.
обычный вариант
При закрытии страницы в браузере, информация не сохраняется.
с помощью свойства
обычный вариант
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> </div> <asp:Label ID="Label1" runat="server" Text="Add notes : "></asp:Label> <asp:TextBox ID="TextBox1" runat="server" Width="375px"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="Add" Width="103px" OnClick="Button1_Click" /> <br /> <br /> <asp:Label ID="Label2" runat="server" Text="Amount added of notes : "></asp:Label> <b> <asp:Label ID="Label3" runat="server" Text=""></asp:Label> </b> <br /> <br /> <asp:Label ID="Label4" runat="server" Text=""></asp:Label> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> </div> <asp:Label ID="Label1" runat="server" Text="Add notes : "></asp:Label> <asp:TextBox ID="TextBox1" runat="server" Width="375px"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="Add" Width="103px" OnClick="Button1_Click" /> <br /> <br /> <asp:Label ID="Label2" runat="server" Text="Amount added of notes : "></asp:Label> <b> <asp:Label ID="Label3" runat="server" Text=""></asp:Label> </b> <br /> <br /> <asp:Label ID="Label4" runat="server" Text=""></asp:Label> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Button1_Click(object sender, EventArgs e) { /*количество сделанных записей*/ int amount = 0; //чтение данных из ViewState object objAmount = ViewState["amount"]; if(objAmount != null) { amount = (int)objAmount; } amount += 1; //запись данных в ViewState ViewState["amount"] = amount; /*текст записей*/ string notes = string.Empty; //чтение данных из ViewState object objNotes = ViewState["notes"]; if(objNotes != null) { notes = (string)objNotes; } notes += TextBox1.Text; //запись данных в ViewState ViewState["notes"] = notes; //очищаем поле ввода TextBox1.Text = string.Empty; //отображаем данные Label3.Text = Convert.ToString(ViewState["amount"]); Label4.Text = Convert.ToString(ViewState["notes"]); } protected void Page_Load(object sender, EventArgs e) { } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Button1_Click(object sender, EventArgs e) { /*количество сделанных записей*/ int amount = 0; //чтение данных из ViewState object objAmount = ViewState["amount"]; if(objAmount != null) { amount = (int)objAmount; } amount += 1; //запись данных в ViewState ViewState["amount"] = amount; /*текст записей*/ string notes = string.Empty; //чтение данных из ViewState object objNotes = ViewState["notes"]; if(objNotes != null) { notes = (string)objNotes; } notes += TextBox1.Text; //запись данных в ViewState ViewState["notes"] = notes; //очищаем поле ввода TextBox1.Text = string.Empty; //отображаем данные Label3.Text = Convert.ToString(ViewState["amount"]); Label4.Text = Convert.ToString(ViewState["notes"]); } protected void Page_Load(object sender, EventArgs e) { } }
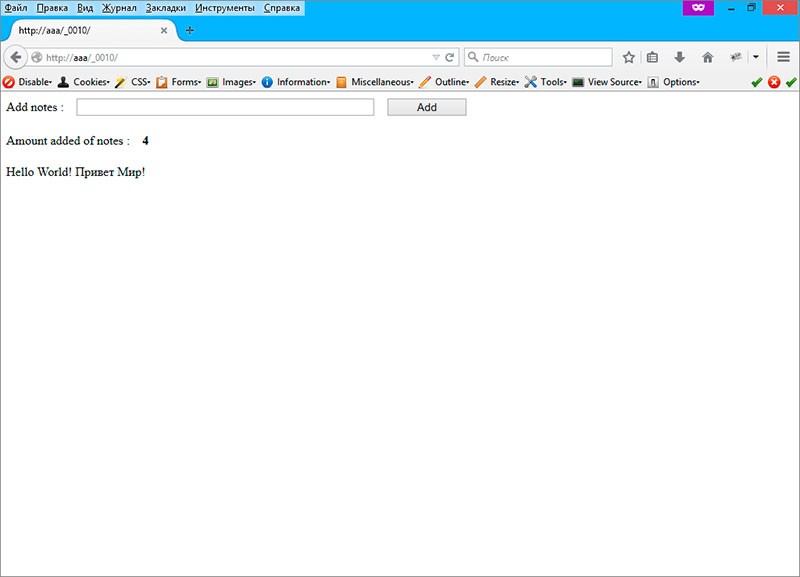
с помощью свойства
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> </div> <asp:Label ID="Label1" runat="server" Text="Add notes : "></asp:Label> <asp:TextBox ID="TextBox1" runat="server" Width="375px"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="Add" Width="103px" OnClick="Button1_Click" /> <br /> <br /> <asp:Label ID="Label2" runat="server" Text="Amount added of notes : "></asp:Label> <b> <asp:Label ID="Label3" runat="server" Text=""></asp:Label> </b> <br /> <br /> <asp:Label ID="Label4" runat="server" Text=""></asp:Label> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> </div> <asp:Label ID="Label1" runat="server" Text="Add notes : "></asp:Label> <asp:TextBox ID="TextBox1" runat="server" Width="375px"></asp:TextBox> <asp:Button ID="Button1" runat="server" Text="Add" Width="103px" OnClick="Button1_Click" /> <br /> <br /> <asp:Label ID="Label2" runat="server" Text="Amount added of notes : "></asp:Label> <b> <asp:Label ID="Label3" runat="server" Text=""></asp:Label> </b> <br /> <br /> <asp:Label ID="Label4" runat="server" Text=""></asp:Label> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { int Amount { set { //запись данных в ViewState ViewState["amount"] = value; } get { //чтение данных из ViewState object objAmount = ViewState["amount"]; if(objAmount != null) { return (int)objAmount; } else { ViewState["amount"] = 0; return 0; } } } string Notes { set { //запись данных в ViewState ViewState["notes"] = value; } get { //чтение данных из ViewState object objNotes = ViewState["notes"]; if(objNotes != null) { return (string)objNotes; } else { ViewState["notes"] = string.Empty; return string.Empty; } } } protected void Button1_Click(object sender, EventArgs e) { Amount += 1; Notes += TextBox1.Text; //очищаем поле ввода TextBox1.Text = string.Empty; //отображаем данные Label3.Text = Convert.ToString(Amount); Label4.Text = Notes; } protected void Page_Load(object sender, EventArgs e) { } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { int Amount { set { //запись данных в ViewState ViewState["amount"] = value; } get { //чтение данных из ViewState object objAmount = ViewState["amount"]; if(objAmount != null) { return (int)objAmount; } else { ViewState["amount"] = 0; return 0; } } } string Notes { set { //запись данных в ViewState ViewState["notes"] = value; } get { //чтение данных из ViewState object objNotes = ViewState["notes"]; if(objNotes != null) { return (string)objNotes; } else { ViewState["notes"] = string.Empty; return string.Empty; } } } protected void Button1_Click(object sender, EventArgs e) { Amount += 1; Notes += TextBox1.Text; //очищаем поле ввода TextBox1.Text = string.Empty; //отображаем данные Label3.Text = Convert.ToString(Amount); Label4.Text = Notes; } protected void Page_Load(object sender, EventArgs e) { } }
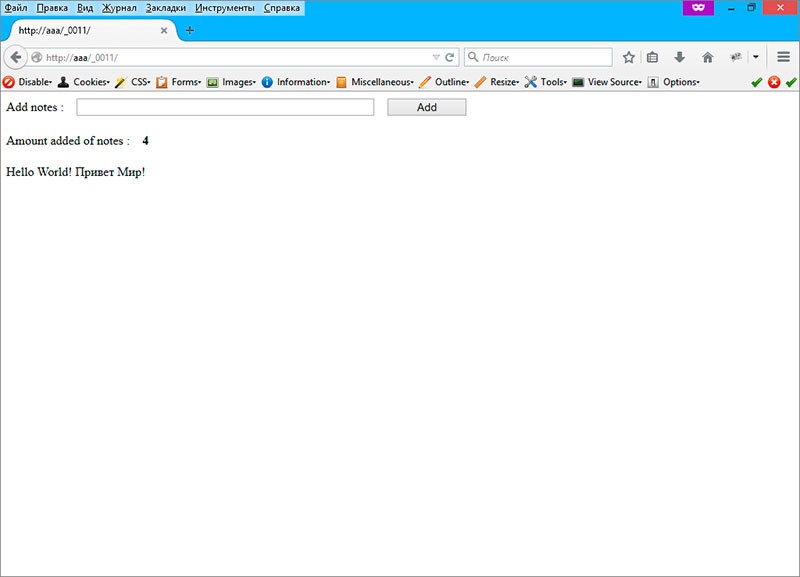