SOAP — протокол передачи данных, который работает поверх протокола HTTP между веб службой и веб сервером
XML — формат передачи документов между веб службой и веб сервером
WSDL — протокол описания данных
UDDI — протокол поиска веб служб в интернете
XML — формат передачи документов между веб службой и веб сервером
WSDL — протокол описания данных
UDDI — протокол поиска веб служб в интернете
создание веб службы
Обязательно нужно выбрать .Net Framework 3.5, потому что в версиях выше 3.5 шаблона веб службы нет.
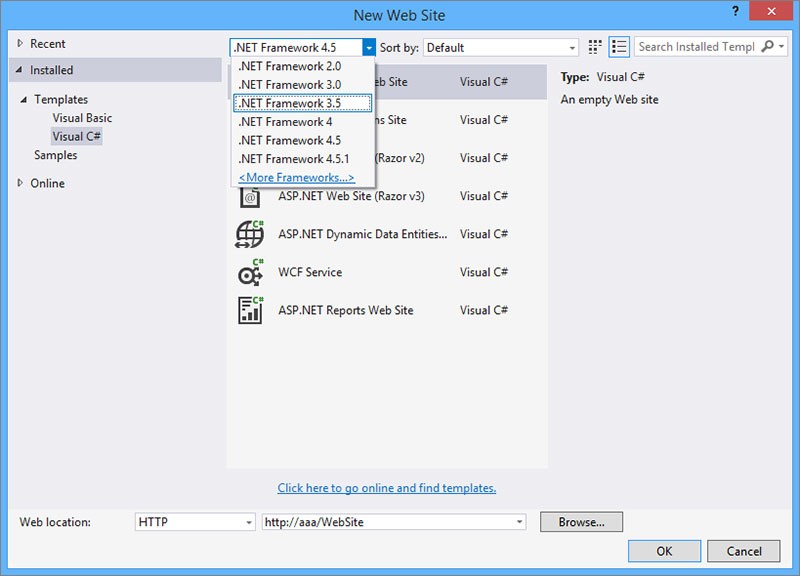
Выберите шаблон ASP.NET Web Service.
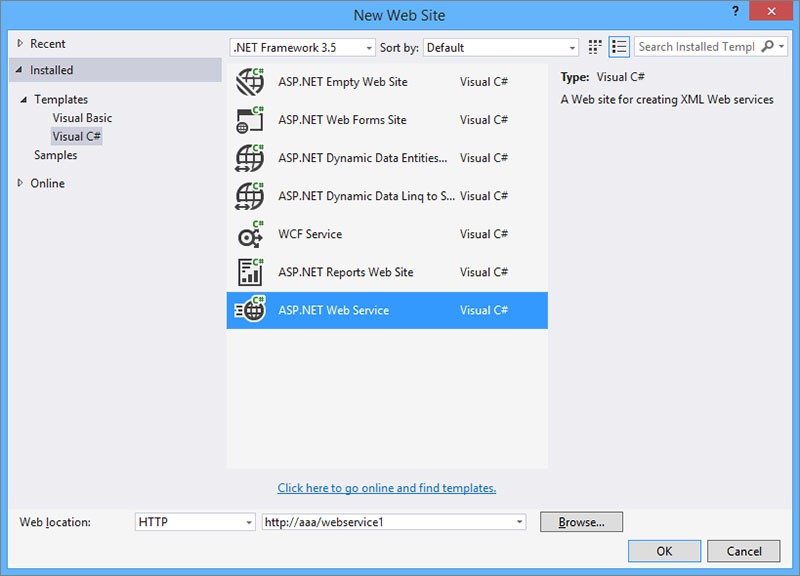
Visual Studio сгенерировала уже готовую веб службу с методом HelloWorld().
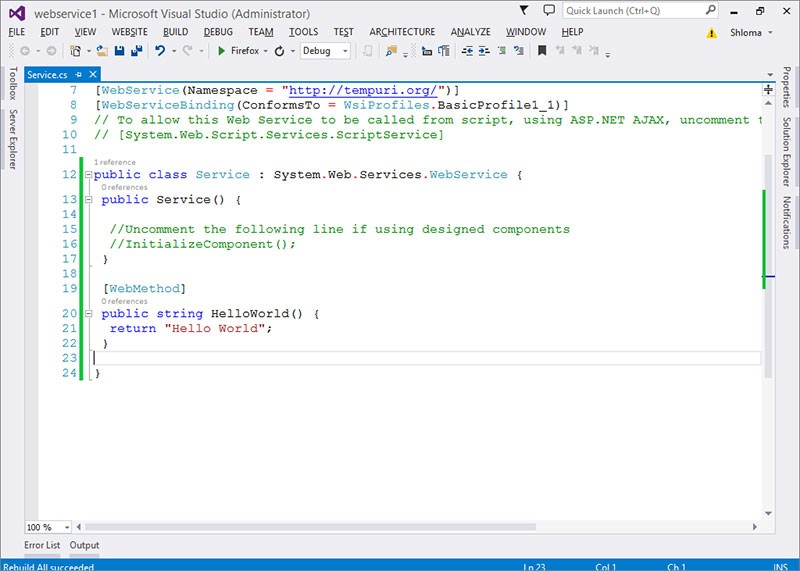
Service.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Services; [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] // To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class Service : System.Web.Services.WebService { public Service() { //Uncomment the following line if using designed components //InitializeComponent(); } [WebMethod] public string HelloWorld() { return "Hello World"; } //создаем класс public class Book { int id; string name; int amount; decimal price; public int ID { set { id = value; } get { return id; } } public string Name { set { name = value; } get { return name; } } public int Amount { set { amount = value; } get { return amount; } } public decimal Price { set { price = value; } get { return price; } } } //создаем метод, обязательно нужно добавить атрибут [WebMethod] [WebMethod] public Book[] GetBooks() { List<Book> arra = new List<Book>(); arra.Add(new Book{ID=1, Name="Азбука", Amount=10, Price=10.25m}); arra.Add(new Book{ID=2, Name="Букварь", Amount=20, Price=20.55m}); arra.Add(new Book{ID=3, Name="Математика", Amount=30, Price=30.75m}); return arra.ToArray(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Services; [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] // To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class Service : System.Web.Services.WebService { public Service() { //Uncomment the following line if using designed components //InitializeComponent(); } [WebMethod] public string HelloWorld() { return "Hello World"; } //создаем класс public class Book { int id; string name; int amount; decimal price; public int ID { set { id = value; } get { return id; } } public string Name { set { name = value; } get { return name; } } public int Amount { set { amount = value; } get { return amount; } } public decimal Price { set { price = value; } get { return price; } } } //создаем метод, обязательно нужно добавить атрибут [WebMethod] [WebMethod] public Book[] GetBooks() { List<Book> arra = new List<Book>(); arra.Add(new Book{ID=1, Name="Азбука", Amount=10, Price=10.25m}); arra.Add(new Book{ID=2, Name="Букварь", Amount=20, Price=20.55m}); arra.Add(new Book{ID=3, Name="Математика", Amount=30, Price=30.75m}); return arra.ToArray(); } }
Запустите код на выполнение.
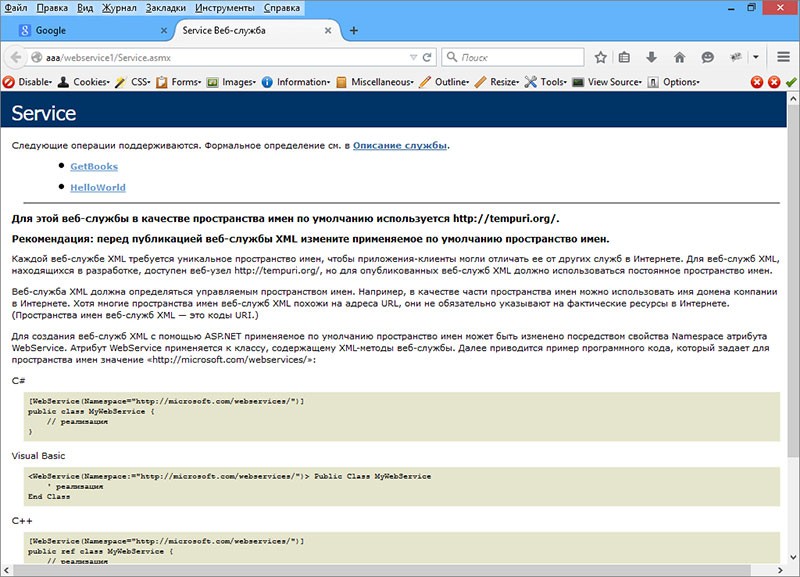
Кликните на созданный метод GetBooks.
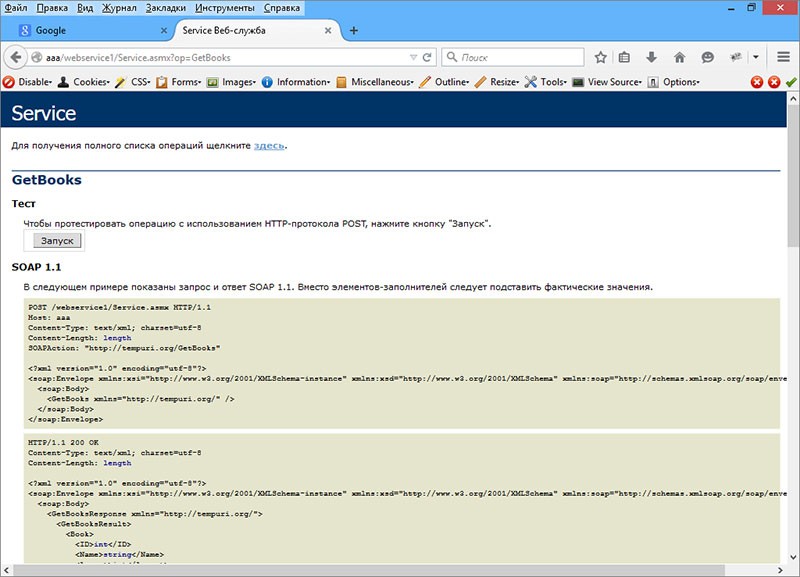
Кликните на копку Запуск. Коллекция класса была преобразована в XML.
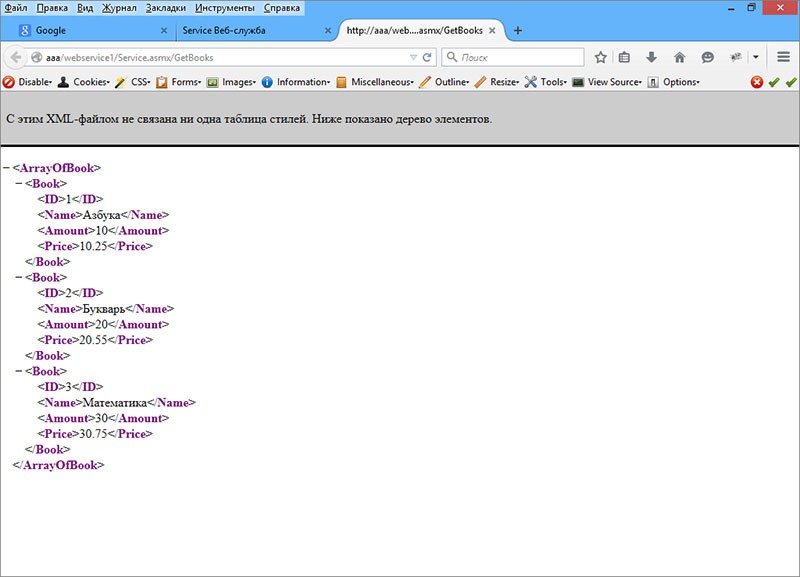
создание веб сайта использующего веб службу
Создайте веб сайт в этом решении.
Сделайте связывание между этим веб сайтом и веб службой.
Скопируйте ссылку веб службы. В моем примере это http://aaa/webservice1/Service.asmx
Добавьте ссылку в веб сайт. Add. Service Reference.
Сделайте связывание между этим веб сайтом и веб службой.
Скопируйте ссылку веб службы. В моем примере это http://aaa/webservice1/Service.asmx
Добавьте ссылку в веб сайт. Add. Service Reference.
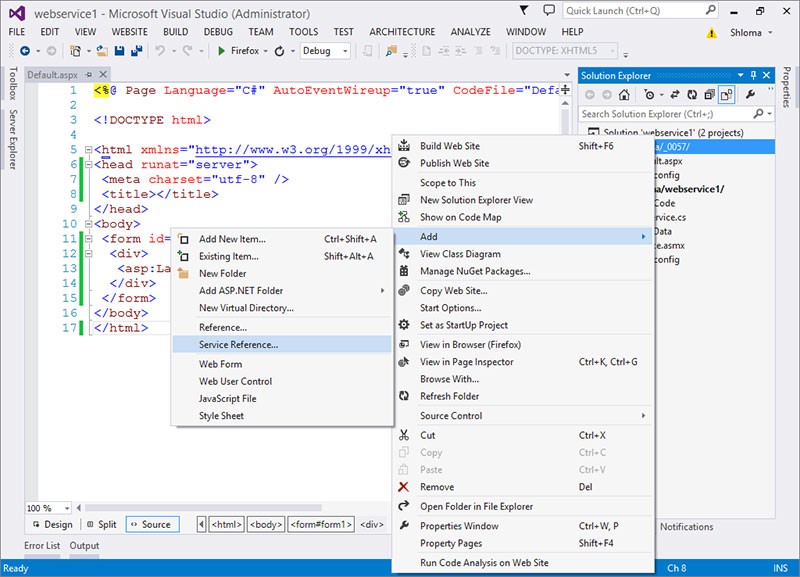
Вставьте URL адрес.
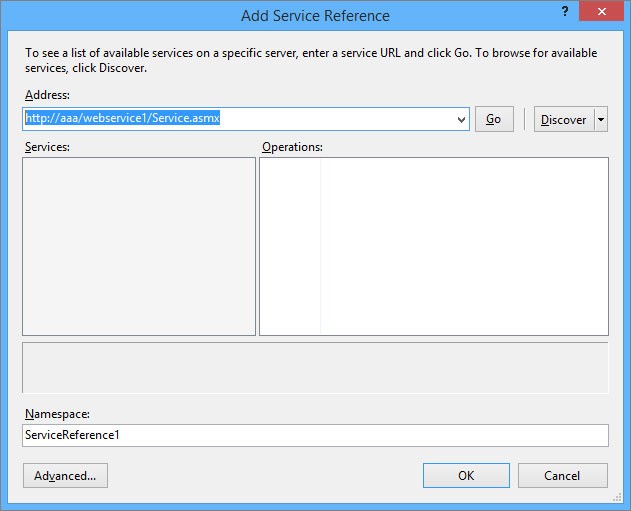
Нажмите GO.
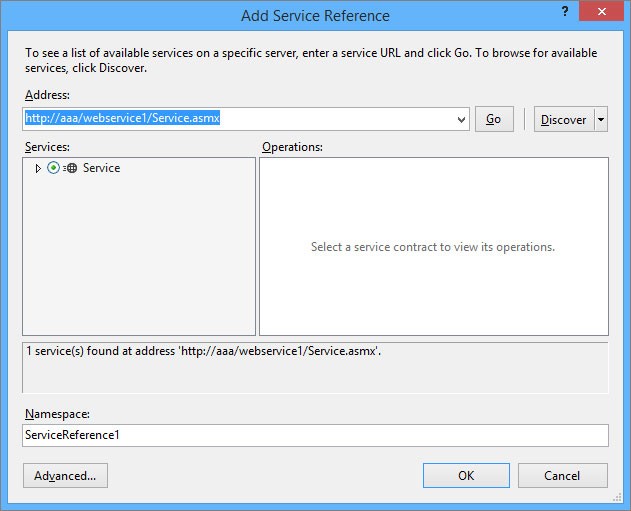
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { //создаем экземпляр объекта службы ServiceReference1.ServiceSoapClient S = new ServiceReference1.ServiceSoapClient(); foreach(var i in S.GetBooks()) { Label1.Text += i.ID + " " + i.Name + " " + i.Amount + " " + i.Price + "<br />"; } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { //создаем экземпляр объекта службы ServiceReference1.ServiceSoapClient S = new ServiceReference1.ServiceSoapClient(); foreach(var i in S.GetBooks()) { Label1.Text += i.ID + " " + i.Name + " " + i.Amount + " " + i.Price + "<br />"; } } }
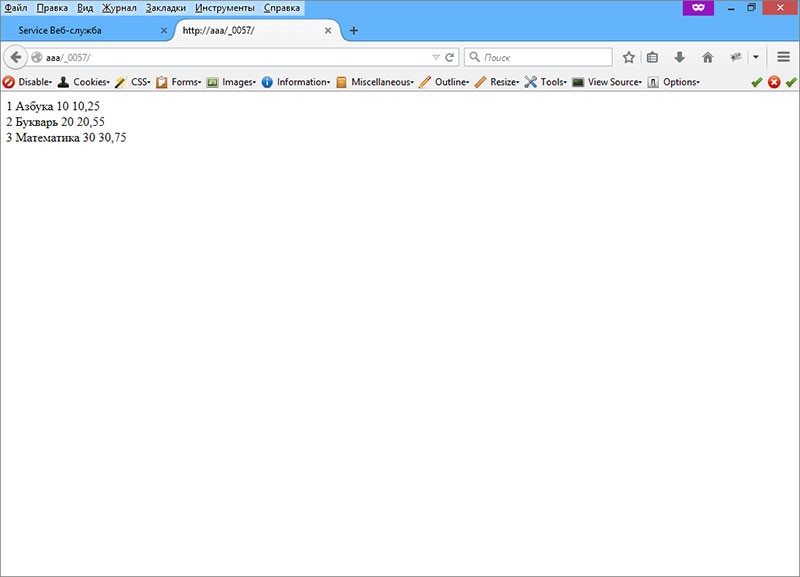