привязка к коллекции
привязка к коллекции объектов
использование всех шаблонов
привязка к базе данных
привязка к коллекции объектов
использование всех шаблонов
привязка к базе данных
привязка к коллекции
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <ItemTemplate> <div> <%-- DataItem - это элемент, который извлекается из коллекции --%> <%# Container.DataItem %> </div> <br /> </ItemTemplate> </asp:Repeater> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <ItemTemplate> <div> <%-- DataItem - это элемент, который извлекается из коллекции --%> <%# Container.DataItem %> </div> <br /> </ItemTemplate> </asp:Repeater> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<string> Colors = new List<string>() { "Red", "Green", "Blue", "Yellow", "Black", "White" }; Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { List<string> Colors = new List<string>() { "Red", "Green", "Blue", "Yellow", "Black", "White" }; Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
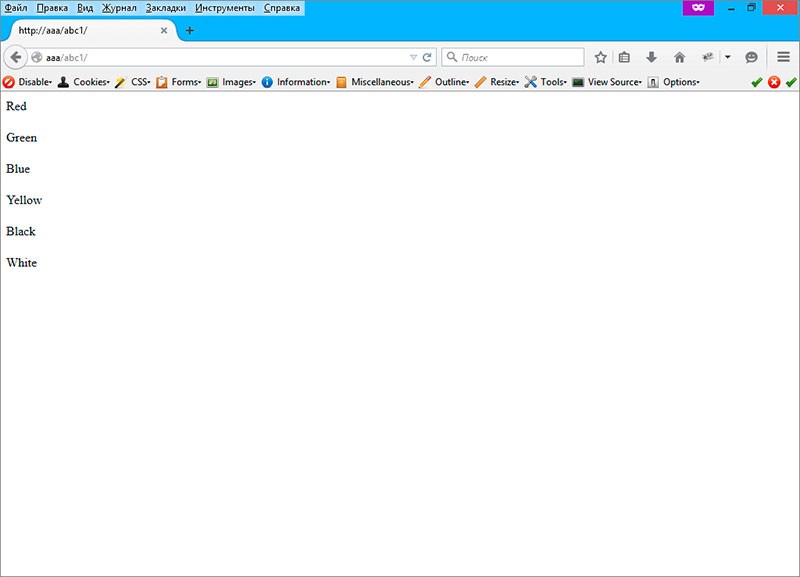
привязка к коллекции объектов
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <ItemTemplate> <div> <%-- метод Eval() с помощью рефлексии ищет указанное свойство --%> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> <br /> </ItemTemplate> </asp:Repeater> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <ItemTemplate> <div> <%-- метод Eval() с помощью рефлексии ищет указанное свойство --%> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> <br /> </ItemTemplate> </asp:Repeater> </div> </form> </body> </html>
Default.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
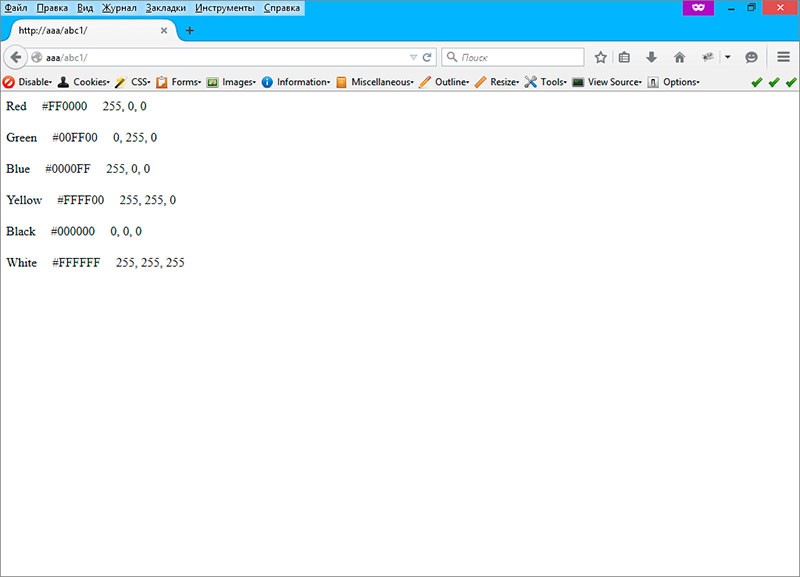
использование всех шаблонов
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> .a { height: 50px; width: 400px; line-height: 50px; text-align: center; background-color: #d3ffce; margin: 5px auto; } .b { height: 50px; width: 400px; line-height: 50px; text-align: center; background-color: #b0e0e6; margin: 5px auto; } .c { height: 1px; width: 400px; background-color: #8a2be2; margin-top: 5px; margin-bottom: 5px; margin: 5px auto; } h2 { text-align: center; color: #800080; } </style> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <HeaderTemplate> <h2>Hello World!</h2> </HeaderTemplate> <ItemTemplate> <div class="a"> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> </ItemTemplate> <SeparatorTemplate> <div class="c"></div> </SeparatorTemplate> <AlternatingItemTemplate> <div class="b"> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> </AlternatingItemTemplate> <FooterTemplate> <h2>Привет Мир!</h2> </FooterTemplate> </asp:Repeater> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta charset="utf-8" /> <title></title> <style> .a { height: 50px; width: 400px; line-height: 50px; text-align: center; background-color: #d3ffce; margin: 5px auto; } .b { height: 50px; width: 400px; line-height: 50px; text-align: center; background-color: #b0e0e6; margin: 5px auto; } .c { height: 1px; width: 400px; background-color: #8a2be2; margin-top: 5px; margin-bottom: 5px; margin: 5px auto; } h2 { text-align: center; color: #800080; } </style> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server"> <HeaderTemplate> <h2>Hello World!</h2> </HeaderTemplate> <ItemTemplate> <div class="a"> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> </ItemTemplate> <SeparatorTemplate> <div class="c"></div> </SeparatorTemplate> <AlternatingItemTemplate> <div class="b"> <%# Eval("Name") %> <%# Eval("Hex") %> <%# Eval("Rgb") %> </div> </AlternatingItemTemplate> <FooterTemplate> <h2>Привет Мир!</h2> </FooterTemplate> </asp:Repeater> </div> </form> </body> </html>
Default.aspx
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { class Color { string name; string hex; string rgb; public Color(string arg0, string arg1, string arg2) { name = arg0; hex = arg1; rgb = arg2; } public string Name { get { return name; } } public string Hex { get { return hex; } } public string Rgb { get { return rgb; } } } protected void Page_Load(object sender, EventArgs e) { List<Color> Colors = new List<Color>(); Colors.Add(new Color("Red", "#FF0000", "255, 0, 0")); Colors.Add(new Color("Green", "#00FF00", "0, 255, 0")); Colors.Add(new Color("Blue", "#0000FF", "255, 0, 0")); Colors.Add(new Color("Yellow", "#FFFF00", "255, 255, 0")); Colors.Add(new Color("Black", "#000000", "0, 0, 0")); Colors.Add(new Color("White", "#FFFFFF", "255, 255, 255")); Repeater1.DataSource = Colors; Repeater1.DataBind(); } }
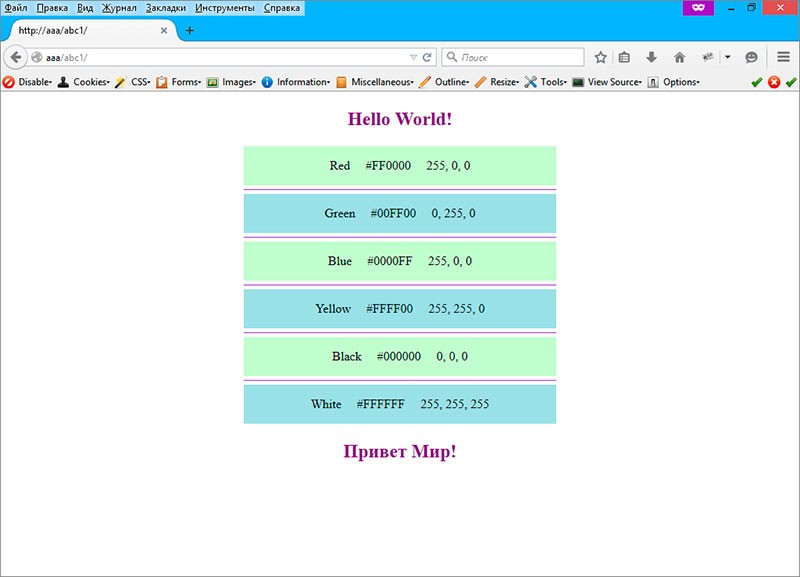
привязка к базе данных
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta /> <title></title> <style> table, td, th{ border: 1px solid #00F; font-size: 20px; border-collapse: collapse; } td, th { padding: 5px; } </style> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server" DataSourceID="SqlDataSource1"> <HeaderTemplate> <table> <tr> <th>ID</th> <th>Name</th> <th>Hex</th> <th>Rgb</th> </tr> </HeaderTemplate> <ItemTemplate> <tr> <td><%# Eval("IDColors") %></td> <td><%# Eval("Name") %></td> <td><%# Eval("Hex") %></td> <td><%# Eval("Rgb") %></td> </tr> </ItemTemplate> <FooterTemplate> </table> </FooterTemplate> </asp:Repeater> <asp:SqlDataSource runat="server" ID="SqlDataSource1" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT * FROM [Colors]"></asp:SqlDataSource> </div> </form> </body> </html>
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta /> <title></title> <style> table, td, th{ border: 1px solid #00F; font-size: 20px; border-collapse: collapse; } td, th { padding: 5px; } </style> </head> <body> <form id="form1" runat="server"> <div> <asp:Repeater ID="Repeater1" runat="server" DataSourceID="SqlDataSource1"> <HeaderTemplate> <table> <tr> <th>ID</th> <th>Name</th> <th>Hex</th> <th>Rgb</th> </tr> </HeaderTemplate> <ItemTemplate> <tr> <td><%# Eval("IDColors") %></td> <td><%# Eval("Name") %></td> <td><%# Eval("Hex") %></td> <td><%# Eval("Rgb") %></td> </tr> </ItemTemplate> <FooterTemplate> </table> </FooterTemplate> </asp:Repeater> <asp:SqlDataSource runat="server" ID="SqlDataSource1" ConnectionString='<%$ ConnectionStrings:ConnectionString %>' SelectCommand="SELECT * FROM [Colors]"></asp:SqlDataSource> </div> </form> </body> </html>
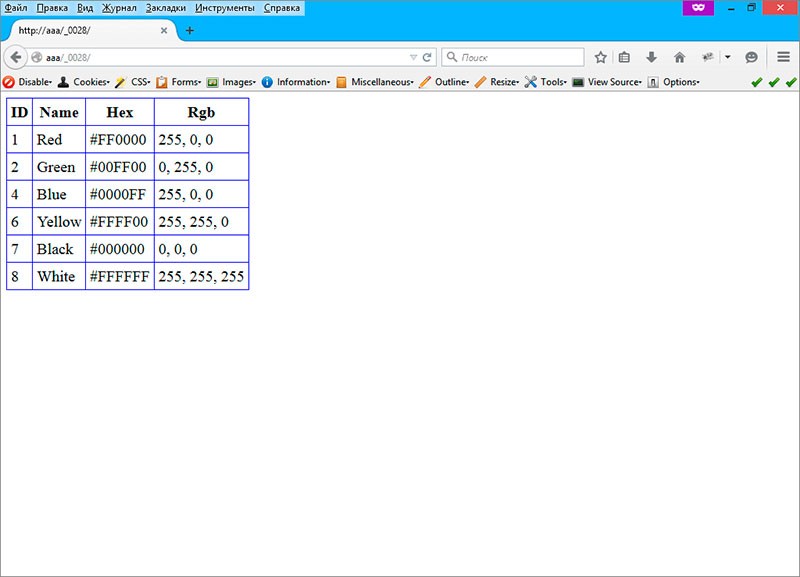